Open a GUI file from another file PyQT
First, you should to name your GUI classes so they have a different name, and not the generic one, so you could distinct them.
Why you would need to do that? Well - simply, because it makes sense - if every class is representing different type of dialog, so it is the different type - and it should be named differently. Some of the names are/may be: QMessageBox
, AboutBox
, AddUserDialog
, and so on.
In Acceuil_start.py (you should rename class in other module, too).
import sys
from PyQt4 import QtCore, QtGui
from Authentification_1 import Ui_Fenetre_auth
from Acceuil_2 import Ui_MainWindow
class Acceuil(QtGui.QMainWindow):
def __init__(self, parent=None):
QtGui.QWidget.__init__(self, parent)
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
if __name__ == "__main__":
app = QtGui.QApplication(sys.argv)
myapp = Acceuil()
myapp.show()
sys.exit(app.exec_())
in the parent class, when you want to create the window, you are close (but it should work in any case):
def authentifier(val): #Slot method
self.Acceuil = Acceuil(self) # You should always pass the parent to the child control
self.Acceuil.show() #???
About parent issue: If your widget/window is creating another widget, setting creator object to be parent is always a good idea (apart from some singular cases), and you should read this to see why is that so:
QObjects organize themselves in object trees. When you create a QObject with another object as parent, it's added to the parent's children() list, and is deleted when the parent is. It turns out that this approach fits the needs of GUI objects very well. For example, a QShortcut (keyboard shortcut) is a child of the relevant window, so when the user closes that window, the shorcut is deleted too.
Edit - Minimal Working Sample
To see what I am trying to tell you, I've built a simple example. You have two classes - MainWindow
and
ChildWindow
. Every class can work without other class by creating separate QApplication
objects. But, if you import ChildWindow
in MainWindow
, you will create ChildWindow
in slot connected to singleshot timer which will trigger in 5 seconds.
MainWindow.py:
import sys
from PyQt4 import QtCore, QtGui
from ChildWindow import ChildWindow
class MainWindow(QtGui.QMainWindow):
def __init__(self, parent=None):
QtGui.QWidget.__init__(self, parent)
QtCore.QTimer.singleShot(5000, self.showChildWindow)
def showChildWindow(self):
self.child_win = ChildWindow(self)
self.child_win.show()
if __name__ == "__main__":
app = QtGui.QApplication(sys.argv)
myapp = MainWindow()
myapp.show()
sys.exit(app.exec_())
ChildWindow.py:
import sys
from PyQt4 import QtCore, QtGui
class ChildWindow(QtGui.QMainWindow):
def __init__(self, parent=None):
QtGui.QWidget.__init__(self, parent)
self.setWindowTitle("Child Window!")
if __name__ == "__main__":
app = QtGui.QApplication(sys.argv)
myapp = ChildWindow()
myapp.show()
sys.exit(app.exec_())
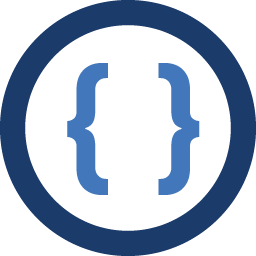
Admin
Updated on June 21, 2022Comments
-
Admin almost 2 years
I've created many GUI interfaces in PyQT using QT Designer, but now I'm trying to open an interface from another one, and I don't know how to do it.. Start.py is the file which run the GUI Interface Authentification_1 and Acceuil_start.py is the file which run the GUI interface Acceuil_2.py, now I want from Start.py to lunch Acceuil_start.py. Do you have any idea about that ? Thank you. Here's my code :
Start.py :
import sys from PyQt4 import QtCore, QtGui from Authentification_1 import Ui_Fenetre_auth from Acceuil_2 import Ui_MainWindow #??? Acceuil_2.py is the file which I want to open class StartQT4(QtGui.QMainWindow): def __init__(self, parent=None): QtGui.QWidget.__init__(self, parent) self.ui = Ui_Fenetre_auth() self.ui.setupUi(self) def authentifier(val): #Slot method self.Acceuil = Acceuil() #??? self.Acceuil.show() #??? if __name__ == "__main__": app = QtGui.QApplication(sys.argv) myapp = StartQT4() myapp.show() sys.exit(app.exec_())
Acceuil_start.py
import sys from PyQt4 import QtCore, QtGui from Authentification_1 import Ui_Fenetre_auth from Acceuil_2 import Ui_MainWindow class StartQT4(QtGui.QMainWindow): def __init__(self, parent=None): QtGui.QWidget.__init__(self, parent) self.ui = Ui_MainWindow() self.ui.setupUi(self) if __name__ == "__main__": app = QtGui.QApplication(sys.argv) myapp = StartQT4() myapp.show() sys.exit(app.exec_())
-
Admin over 11 yearsThank you. Is The parent class, the class which should open the other one ? and why should I change the name of the class in Acceuil_start.py ?
-
Admin over 11 yearsNo, it gives me this error : NameError: global name 'Acceuil' is not defined for :
self.Acceuil = Acceuil(self)
-
Nemanja Boric over 11 yearsDon't forget to import that class:
from Acceuil_start import Acceuil
-
Admin over 11 yearsit gives me :
NameError: global name 'self' is not defined
-
Admin over 11 yearsThank you. it gives me
AttributeError: 'Acceuil' object has no attribute 'exec_'
-
Admin over 11 yearsI want to do like in Java, we instanciate the window like :
Acceuil a = new Acceuil(); a.show();
and the Acceuil() class display it's window -
Nemanja Boric over 11 years@Mehdi You are doing something wrong. I will edit my text to provide minimal working sample.
-
Nemanja Boric over 11 yearsChange
def authentifier(val):
todef authentifier(self):
. -
Nemanja Boric over 11 years@Mehdi I've included simple example which should get you started.