The event loop is already running
I think the problem is with your start.py file. You have a function refreshgui which re imports start.py
import will run every part of the code in the file. It is customary to wrap the main functionality in an ''if __name__ == '__main__': to prevent code from being run on import.
The error you are getting happens everytime you have more than one QApplication or QCoreApplication.
if __name__ == '__main__': # only executes the below code if it python has run it as the main
app = QtGui.QApplication(sys.argv) # before this was getting called twice
myapp = StartQT4()
myapp.show()
threadhandler.variablesinitialize()
threadhandler.functionsinitialize()
timer = QtCore.QTimer()
timer.timeout.connect(functions.refreshgui) # I believe this re-imports start.py which calls all of this code again.
timer.start(1000)
sys.exit(app.exec_())
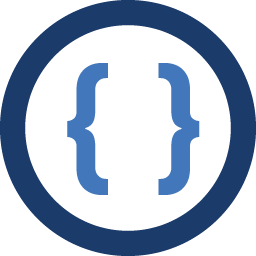
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I have the following 5 files:
gui.py
# -*- coding: utf-8 -*- from PyQt4 import QtCore, QtGui try: _fromUtf8 = QtCore.QString.fromUtf8 except AttributeError: def _fromUtf8(s): return s try: _encoding = QtGui.QApplication.UnicodeUTF8 def _translate(context, text, disambig): return QtGui.QApplication.translate(context, text, disambig, _encoding) except AttributeError: def _translate(context, text, disambig): return QtGui.QApplication.translate(context, text, disambig) class Ui_MainWindow(object): def setupUi(self, MainWindow): MainWindow.setObjectName(_fromUtf8("MainWindow")) MainWindow.resize(385, 365) sizePolicy = QtGui.QSizePolicy(QtGui.QSizePolicy.Preferred, QtGui.QSizePolicy.Preferred) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(MainWindow.sizePolicy().hasHeightForWidth()) MainWindow.setSizePolicy(sizePolicy) MainWindow.setMinimumSize(QtCore.QSize(385, 365)) MainWindow.setMaximumSize(QtCore.QSize(385, 365)) self.centralwidget = QtGui.QWidget(MainWindow) self.centralwidget.setEnabled(True) self.centralwidget.setMinimumSize(QtCore.QSize(385, 318)) self.centralwidget.setMaximumSize(QtCore.QSize(385, 318)) self.centralwidget.setObjectName(_fromUtf8("centralwidget")) self.verticalLayout = QtGui.QVBoxLayout(self.centralwidget) self.verticalLayout.setSizeConstraint(QtGui.QLayout.SetDefaultConstraint) self.verticalLayout.setObjectName(_fromUtf8("verticalLayout")) self.textEdit = QtGui.QTextEdit(self.centralwidget) self.textEdit.setObjectName(_fromUtf8("textEdit")) self.verticalLayout.addWidget(self.textEdit) self.pushButton_1 = QtGui.QPushButton(self.centralwidget) self.pushButton_1.setObjectName(_fromUtf8("pushButton_1")) self.verticalLayout.addWidget(self.pushButton_1) self.pushButton_2 = QtGui.QPushButton(self.centralwidget) self.pushButton_2.setObjectName(_fromUtf8("pushButton_2")) self.verticalLayout.addWidget(self.pushButton_2) self.pushButton_3 = QtGui.QPushButton(self.centralwidget) self.pushButton_3.setObjectName(_fromUtf8("pushButton_3")) self.verticalLayout.addWidget(self.pushButton_3) MainWindow.setCentralWidget(self.centralwidget) self.menubar = QtGui.QMenuBar(MainWindow) self.menubar.setEnabled(False) self.menubar.setGeometry(QtCore.QRect(0, 0, 385, 24)) self.menubar.setObjectName(_fromUtf8("menubar")) MainWindow.setMenuBar(self.menubar) self.statusbar = QtGui.QStatusBar(MainWindow) self.statusbar.setEnabled(False) self.statusbar.setObjectName(_fromUtf8("statusbar")) MainWindow.setStatusBar(self.statusbar) self.retranslateUi(MainWindow) QtCore.QMetaObject.connectSlotsByName(MainWindow) def retranslateUi(self, MainWindow): MainWindow.setWindowTitle(_translate("MainWindow", "MainWindow", None)) self.pushButton_1.setText(_translate("MainWindow", "Start a thread", None)) self.pushButton_2.setText(_translate("MainWindow", "Toggle Timer", None)) self.pushButton_3.setText(_translate("MainWindow", "Exit", None))
functions.py
import variables, logging def initialize(): variables.lock.acquire() try: pass finally: variables.lock.release() def refreshgui(): import start if variables.threadcounter != 0: start.myapp.ui.textEdit.setText(variables.globalstring + ' with ' + str(variables.threadcounter) + ' running threads' + '\nCounter: ' + str(variables.counter) + ' seconds') else: start.myapp.ui.textEdit.setText('String not submitted' + ' with ' + str(variables.threadcounter) + ' running threads' + '\nCounter: ' + str(variables.counter) + ' seconds') variables.counter += 1
threadhandler.py
import variables, functions, threading def variablesinitialize(): t = threading.Thread(name='Variables initialize', target=variables.initialize) t.start() t.join() def functionsinitialize(): t = threading.Thread(name='Functions initialize', target=functions.initialize) t.start() t.join()
variables.py
import logging, threading def initialize(): global globalstring, counter, threadcounter, lock lock = threading.Lock() lock.acquire() try: logging.basicConfig(level=logging.INFO, format='%(asctime)s (%(threadName)-2s) %(message)s', datefmt='%d/%m/%Y %H:%M:%S') globalstring = 'No Success' counter = 0 threadcounter = 0 finally: lock.release()
and start.py
# -*- coding: utf-8 -*- import sys from PyQt4 import QtCore, QtGui from gui import Ui_MainWindow import threadhandler, functions class StartQT4(QtGui.QMainWindow): def __init__(self, parent=None): QtGui.QWidget.__init__(self, parent) self.ui = Ui_MainWindow() self.ui.setupUi(self) QtCore.QObject.connect(self.ui.pushButton_1,QtCore.SIGNAL("clicked()"), exit) QtCore.QObject.connect(self.ui.pushButton_2,QtCore.SIGNAL("clicked()"), exit) QtCore.QObject.connect(self.ui.pushButton_3,QtCore.SIGNAL("clicked()"), exit) app = QtGui.QApplication(sys.argv) myapp = StartQT4() myapp.show() threadhandler.variablesinitialize() threadhandler.functionsinitialize() timer = QtCore.QTimer() timer.timeout.connect(functions.refreshgui) timer.start(1000) sys.exit(app.exec_())
After executing start.py i get the message: "QCoreApplication:exec: The event loop is already running"
I figured out, that it has something to do with the timer
timer = QtCore.QTimer() timer.timeout.connect(functions.refreshgui) timer.start(1000)
out of start.py and the function
def refreshgui(): import start if variables.threadcounter != 0: start.myapp.ui.textEdit.setText(variables.globalstring + ' with ' + str(variables.threadcounter) + ' running threads' + '\nCounter: ' + str(variables.counter) + ' seconds') else: start.myapp.ui.textEdit.setText('String not submitted' + ' with ' + str(variables.threadcounter) + ' running threads' + '\nCounter: ' + str(variables.counter) + ' seconds') variables.counter += 1
out of functions.py
Could someone explain to me what the problem is? I don't know what's wrong with the code...
-
ekhumoro almost 9 yearsThe key point is that
start.py
doesn't get added tosys.modules
when it's run as the__main__
script. So if it's later imported as a normal module, its top-level code will be executed again. This is unlike all the other imports, which immediately get cached insys.modules
, and so won't get executed again if re-imported.