Open a modal or a dialogue after navigation in Flutter
879
It's my test code with your code.
It works well.
import 'dart:async';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
void initState() {
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: AfterSplash(),
floatingActionButton: FloatingActionButton(
onPressed: () {},
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
class AfterSplash extends StatefulWidget {
@override
_AfterSplashState createState() => _AfterSplashState();
}
class _AfterSplashState extends State<AfterSplash> {
void initState() {
super.initState();
Timer.run(() {
showDialog(
context: context,
builder: (_) => AlertDialog(title: Text("Dialog title")),
);
});
}
@override
Widget build(BuildContext context) {
return opacityLogoTitle();
}
}
Widget opacityLogoTitle() {
return Scaffold(
body: Opacity(
opacity: 0.5,
child: Scaffold(
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Center(
child: Padding(
padding: const EdgeInsets.all(20.0),
child: Container(child: Text('asdf')),
),
),
Text(
'Sample App',
style: TextStyle(
fontFamily: 'SF Pro Display',
fontSize: 60,
color: Color.fromRGBO(105, 121, 248, 1),
),
),
],
),
),
),
);
}
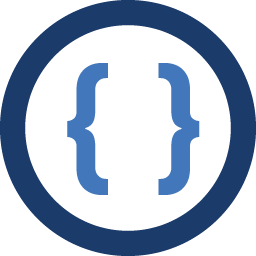
Author by
Admin
Updated on December 24, 2022Comments
-
Admin over 1 year
After calling Navigator pushReplacement, I arrive at a screen where I'd like to open a modal or a dialog automatically after the screen loads. I'm trying to do that using Timer.run inside initState() but it doesn't work, it doesn't show any errors as well. Could anyone help me understand what am I missing here?
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'dart:async'; class AfterSplash extends StatefulWidget { @override _AfterSplashState createState() => _AfterSplashState(); } class _AfterSplashState extends State<AfterSplash> { void initState() { super.initState(); Timer.run(() { showDialog( context: context, builder: (_) => AlertDialog(title: Text("Dialog title")), ); }); } @override Widget build(BuildContext context) { return opacityLogoTitle(); } } Widget opacityLogoTitle() { return Scaffold( body: Opacity( opacity: 0.5, child: Scaffold( body: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Center( child: Padding( padding: const EdgeInsets.all(20.0), child: Container( child: Image(image: AssetImage('assets/images/main.png')), ), ), ), Text( 'Sample App', style: TextStyle( fontFamily: 'SF Pro Display', fontSize: 60, color: Color.fromRGBO(105, 121, 248, 1), ), ), ], ), ), ), ); }
-
Abhijith over 3 yearsI have tested this code with dartpad ,like user KuKu said it works fine@gogolism
-
-
Admin over 3 yearsDoes work now. Thanks for the code. Another problem I found in my code was the inconsistent use of Material & Cupertino widgets. As I replaced all Cupertino with Material ones, it started working.