Owl carousel still transitions when only 1 slide in carousel
Solution 1
For the new beta version see below
Owl Carousel 1.3.2
In this version, it doesn't seem that the plugin has a setting for this. You can do this on your own, either before or after the plugin has been initialized.
Option 1 - before plugin initialization
The best approach would be for you to detect this situation before initializing the plugin altogether.
For example:
$(document).ready( function () {
$owlContainer = $('#owl-demo');
$owlSlides = $owlContainer.children('div');
// More than one slide - initialize the carousel
if ($owlSlides.length > 1) {
$owlContainer.owlCarousel({
// options go here
});
// Only one slide - do something else
} else {
//...
}
});
Option 2 - after plugin initialization
It might be the case that you're relying on the plugin to also style and dynamically adjust the item. In this situation, you can detect there's only one slide after initialization and then stop the transitions. You can do this with the afterInit
callback and the stop
method.
For example:
$(document).ready( function () {
$('#owl-demo').owlCarousel({
// other options go here
//...,
afterInit: function() {
$owlContainer = $('#owl-demo');
$owlSlides = $owlContainer.children('div');
// Only one slide - stop the carousel
if ($owlSlides.length == 1) {
$owlContainer.stop();
}
}
});
});
Owl Carousel 2 Beta
In this new revamped version of the plugin, the API has been completely replaced. The same approaches are still possible, but the implementation is a little different.
Option 1 - before plugin initialization
The new API now includes an option named autoplay
, which allows to directly control the carousel's behavior once it's initialized. By default this option is set to false
, but we can set it as we please according to the number of slides.
For example:
$(document).ready( function () {
$owlContainer = $('#owl-demo');
$owlSlides = $owlContainer.children('div');
owlAutoPlay = $owlSlide.length > 1;
$('#owl-demo').owlCarousel({
// other options go here
//...,
autoplay: owlAutoPlay
}
});
Alternatively, according to the number of slides, we can also choose to not initialize the plugin altogether, as we did in the previous version (see option 1 above).
Option 2 - after plugin initialization
As in the previous version, if you must initialize the plugin (and if you must have the autoplay
option set to true)
, you can also stop the carousel after initialization. However, in this version the initialization callback option is now named onInitialized
. Also, there's no direct .stop()
method. Instead you will need to trigger the autoplay.stop.owl
event of the carousel.
For example:
$(document).ready( function () {
$('#owl-demo').owlCarousel({
// Other options go here
// ...,
onInitialized: function() {
if ($('#owl-demo').children().length == 1) {
$('#owl-demo').trigger('autoplay.stop.owl');
}
}
});
});
Solution 2
You can check if there is 1 item in your carousel and activate 'autoplay' or not. In your case it will be like below.
$(document).ready(function () {
$("#owl-demo").owlCarousel({
navigation: false,
stopOnHover: true,
paginationSpeed: 1000,
goToFirstSpeed: 2000,
autoHeight : true,
transitionStyle:"fade",
singleItem: true
autoPlay: $("#owl-demo > div").length > 1 ? 5000 : false
});
});
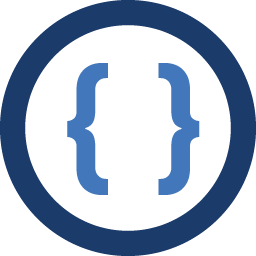
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I wonder if anyone could help with this. I am using carousel in a CMS and would like the ability for the customer to sometimes only have 1 slide if they so wish. However if only 1 slide is there the fade transition still occurs so it basically transitions to itself. Is there any way to stop the carousel transitioning when there is only 1 slide? I am surprised that this is not a built in function as it has been with other carousels I have used.
<div id="owl-demo" class="owl-carousel"> <div class="item"> <h2><umbraco:Item field="bigMessage" stripParagraph="true" convertLineBreaks="true" runat="server" /></h2> <p><umbraco:Item field="messageSubtext" stripParagraph="true" convertLineBreaks="true" runat="server" /></p> <umbraco:image runat="server" field="bannerImage" /> </div> </div> <script src="/owl-carousel/owl.carousel.js"></script> <style> #owl-demo .item img{ display: block; width: 100%; height: auto; } </style> <script> $(document).ready(function() { $("#owl-demo").owlCarousel({ navigation: false, stopOnHover: true, paginationSpeed: 1000, autoPlay: 5000, goToFirstSpeed: 2000, autoHeight : true, transitionStyle:"fade", singleItem: true // "singleItem:true" is a shortcut for: // items : 1, // itemsDesktop : false, // itemsDesktopSmall : false, // itemsTablet: false, // itemsMobile : false }); }); </script>