Parse a String in C
Solution 1
OK, you're either lazy, or stuck, assuming stuck.
You need a function with a signature something like
int ReplaceCharInString(char* string, char charToFind, char charThatReplaces)
{
}
Inside the function you need
- To declare an integer to count the occurrences
- A loop that moves from the start of the string to it's end
- inside the loop, an if statement to check is the current char the charToFind,
- statements to increment the count of occurrences and perform the replacement
- After the loop, you need to return the count of occurrences
Solution 2
This function will take a string, replace every 'e' with '3', and return the number of times it performed the substitution. It's safe, it's clean, it's fast.
int e_to_three(char *s)
{
char *p;
int count = 0;
for (p = s; *p; ++p) {
if (*p == 'e') {
*p = '3';
count++;
}
}
return count;
}
Solution 3
Here's a shell to get you started. Ask here if you need any help.
#include <string.h>
#include <stdio.h>
int main(){
const char* string = "hello world";
char buffer[256];
int e_count = 0;
char* walker;
// Copy the string into a workable buffer
strcpy(buffer,string);
// Do the operations
for(walker=buffer;*walker;++walker){
// Use *walker to read and write the current character
}
// Print it out
printf("String was %s\nNew string is %s\nThere were %d e's\n",string,buffer,e_count);
}
Solution 4
Some of you guys are starting in the middle.
A better start would be
char *string = "hello world";
Assert(ReplaceCharInString(string, 'e', '3') == 1);
Assert(strcmp(string, "h3llo world") == 0);
Solution 5
In general, it's better use a standard library function rather than rolling your own. And, as it just so happens, there is a standard library function that searches a string for a character and returns a pointer to it. (It deals with a string, so look among the functions that have the prefix "str") (The library function will almost certainly be optimized to use specialized CPU opcodes for the task, that hand written code would not)
Set a temp pointer (say "ptr") to the start of the string.
In a loop, call the function above using ptr as the parameter, and setting it to the return value.
Increment a counter.
Set the character at the pointer to "3" break when 'e' is not found.
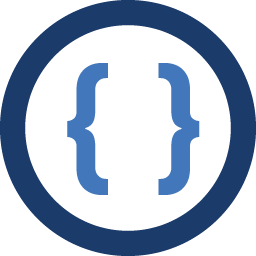
Admin
Updated on October 13, 2020Comments
-
Admin over 3 years
Using just C
I would like to parse a string and:
- count the occurrences of a character in a string (for example, count all the
'e'
s in a passed in string) - Once counted (or even as I am counting) replace the e's with 3's
- count the occurrences of a character in a string (for example, count all the
-
Rich almost 15 yearsBecause it's a solution for a homework question, I guess (no I wasn't the downvoter). Posting actually working code solutions for homework just encourages bad behaviour on part of lazy people :)
-
paxdiablo almost 15 yearsUpvoting since the homework tag wasn't in the original question, it was added by someone else.
-
Admin almost 15 yearsWhether the homework tag is there or not, it isn't helpful to anyone (includingb the questioner) to give answers to these "post da codez pleez" type questions.
-
Kapil D almost 15 yearsalso, the solution is wrong I suppose what is the passed string is char * s = "Name"; in that case *p=3 will give an error as the "Name" string constant
-
Alexander H almost 15 yearsThis is presumably a suggestion to write the test first. It is interesting but I'm not sure that it's the best technique for writing with pointer-heavy code in C, because of the unpredictable and unrepeatable effects of incomplete code.
-
David Sykes almost 15 years@Edmund I'd be interested to know why that might be the case. At the very least the tests specify what the routine is to do, and confirms it does so
-
Alexander H almost 15 yearsI think the tests are useful, but I think the pain of writing them and getting them working from scratch is more in C than in non-pointery languages and hence the benefit of TDD is diminished. If it fails non-gracefully, it's much harder to know why. If it passes, it might still have disrupted things for other tests.
-
David Sykes almost 15 yearsI can't say that has been my experience, but maybe it depends what sort of code is being written
-
Jonathan Leffler almost 15 yearsWhy did the blank get removed? And what are Assert() and ReplaceCharInString()? They are not ISO standard C functions.
-
David Sykes almost 15 years@Jonathan: typo fixed, thanks. ReplaceCharInString is the function to be written, name suggested by Binary Worrier. Assert is part of the chosen test framework
-
Anatoly Ivanov almost 15 yearsI've done some TDD in C, and it works perfectly fine. I think if you've worked in C, you learn to be careful with NULL and uninitialized local variables. You'll do a lot of "AssertNotNull()" in your tests. Then you mostly worry about infinite loops and walking off the end of buffers. The former locks up your test suite in any language; the latter is a crash in C instead of an exception, but isn't really that common.
-
Dietrich Epp almost 15 yearsReply to let-them-c: char * s = "Name"; should be flagged as an error by your compiler, unless you turn the errors off.