Passing input from html to python and back
Do you have a web server like apache setup this is running on?
If you don't I'm not sure this will work so you may want to have a look at Mamp To allow it to execute your python script you will also need to edit the httpd.conf
file
From:
<Directory />
Options Indexes FollowSymLinks
AllowOverride None
</Directory>
To:
<Directory "/var/www/cgi-bin">
Options +ExecCGI
AddHandler cgi-script .cgi .py
Order allow,vdeny
Allow from all
</Directory>
Alternatively
If you simply want to make an actual HTML file without setting up a server a very basic but crude way of doing this would be to simply write everything to a HTML file you create like:
fo.write("Content-type:text/html\r\n\r\n")
fo.write("<html>")
fo.write("<head>")
fo.write("<title>Hello - Second CGI Program</title>")
fo.write("</head>")
fo.write("<body>")
fo.write("<h2>Your name is {}. {} {}</h2>".format("last_name", "first_name", "last_name"))
fo.write("</body>")
fo.write("</html>")
fo.close()
Which will create a HTML document called yourfile.html
in the same directory as your python project.
I don't recommend doing this, but I realise since it's an assignment you may not have the choice to use libraries. In case you, are a more elegant way would be to use something like yattag which will make it much more maintainable.
To copy the Hello World example from their website.
from yattag import Doc
doc, tag, text = Doc().tagtext()
with tag('h1'):
text('Hello world!')
print(doc.getvalue())
Update:
Another alternative if you don't have a local web server setup is to use Flask as your web server. You'll need to structure your project like:
/yourapp
basic_example.py
/static/
/test.css
/templates/
/test.html
Python:
__author__ = 'kai'
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def index():
return render_template('test.html')
@app.route('/hello', methods=['POST'])
def hello():
first_name = request.form['first_name']
last_name = request.form['last_name']
return 'Hello %s %s have fun learning python <br/> <a href="/">Back Home</a>' % (first_name, last_name)
if __name__ == '__main__':
app.run(host = '0.0.0.0', port = 3000)
HTML:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="static/test.css">
<title>
CV - Rogier
</title>
</head>
<body>
<h3>
Study
</h3>
<p>
At my study we learn Python.<br>
This is a sall example:<br>
<form action="/hello" method="post">
First Name: <input type="text" name="first_name"> <br />
Last Name: <input type="text" name="last_name" />
<input type="submit" name= "form" value="Submit" />
</form>
</p>
</body>
</html>
CSS (If you want to style your form?)
p {
font-family: verdana;
font-size: 20px;
}
h2 {
color: navy;
margin-left: 20px;
text-align: center;
}
Made a basic example based on your question here Hopefully this helps get you on the right track, good luck.
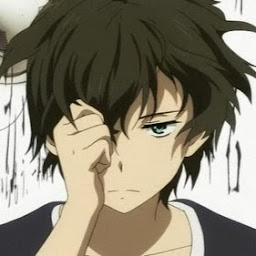
RnRoger
Updated on November 30, 2020Comments
-
RnRoger over 3 years
I need to make a webpage for an assignment, it doesn't have to be uploaded to the web, I am just using a local .html file. I did some reading up and came up with the following html and python:
<!DOCTYPE html> <html> <head> <title> CV - Rogier </title> </head <body> <h3> Study </h3> <p> At my study we learn Python.<br> This is a sall example:<br> <form action="/cgi-bin/cvpython.py" method="get"> First Name: <input type="text" name="first_name"> <br /> Last Name: <input type="text" name="last_name" /> <input type="submit" value="Submit" /> </form> </p> </body> </html>
Python:
import cgi import cgitb #found this but isn't used? form = cgi.FieldStorage() first_name = form.getvalue('first_name').capitalize() last_name = form.getvalue('last_name').capitalize() print ("Content-type:text/html\r\n\r\n") print ("<html>") print ("<head>") print ("<title>Hello - Second CGI Program</title>") print ("</head>") print ("<body>") print ("<h2>Your name is {}. {} {}</h2>".format(last_name, first_name, last_name)) print ("</body>") print ("</html>")
However this just gives the prints as text and not as a proper html file with the 1 line that I want.