Passing params in React Navigation 5
Solution 1
I solved this problem by updating the state in shouldComponentUpdate().
This bug was due to the state not being updated as the screen remained mounted.
This was possible because of ravirajn22 on GitHub who explained to me the reasoning behind this bug and a possible solution.
Link to the answer: https://github.com/react-navigation/react-navigation/issues/6863
Solution 2
/* 1. Navigate to the Details route with params */
onPress={() => {
navigation.navigate('Details', {
itemId: 86,
otherParam: 'anything you want here',
});
}}
/* 2. Get the param */
const DetailsScreen = ({ route, navigation }) => {
const { itemId } = route.params;
const { otherParam } = route.params;
return (
<View></View>
);
};
for more info: https://reactnavigation.org/docs/params/
Solution 3
As it is stated Here, you can do it like this:
navigation.navigate('otherStackName', {
screen: 'destinationScreenName',
params: { user: 'jane' },
});
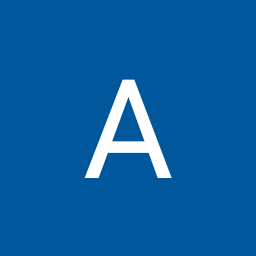
Comments
-
Ali almost 2 years
I'm trying to pass a few params between a Tab Navigator. Below is the structure of my program. In bold are the routes
App(Tab Navigator): { Main(stack) & Filter(screen) }
Main(Stack Navigator): { Home(screen) & MediaDetails(screen) }
I have a button on the screen associated with Filter which has an onPress() function. I'm passing a few params(but let's only consider the param to for the sake of this question).
this.props.navigation.navigate('Home', { to: this.state.to, }
Now in the screen associated with Home, I'm reading the param inside the state like this:
state = { to: this.props.route.params.to }
Inside App.js, I've set the initial value of to to be '2020' like this:
<Stack.Screen name="Home" component={HomeScreen} initialParams={{ to: '2020' }} />
The initial value is indeed set to 2020. I press the button. Let's say I'm setting to to 1900. Just before this.props.navigation.navigate executes, I console.log(this.state.to) the value and it is indeed updated to 1900. However, as the screen changes to Home, the value reverts back to 2020(observed via console.log)
Could someone point out the cause for this spooky behavior? I've been trying to debug this for many hours with no luck. React Navigation 5 is pretty new as well so couldn't find anything similar online. Any help will be greatly appreciated. Thank you for reading all the way.
Edit: Issue has been resolved and full code has been removed!
-
satya164 about 4 yearsCan you post full code?
-
Ali about 4 yearsThanks for showing interest. I've included the full code now.
-
Siraj Alam about 4 yearsUse debugger instead of console.log stackoverflow.com/questions/11284663/…
-
Ali about 4 yearsTried that and also rendering. Doesn't work!
-
-
Variag about 4 yearscan you post that answer or at least a link to it?
-
Ali about 4 yearsI’ve posted the link now. Thank you!
-
pavan kvch over 3 yearsif it is class Component how to get param