The action POP with payload was not handled by any navigator
react-native
react-navigation
react-navigation-stack
react-navigation-v5
react-navigation-bottom-tab
10,916
Solution 1
if(navigation.canGoBack()) {
navigation.dispatch(StackActions.pop(1));
}
see https://github.com/react-navigation/react-navigation/issues/7814#issuecomment-599921016
Solution 2
Error Cause: goBack() or pop() is getting called multiple times. Sometimes onPress event gets called many times. You can check by adding console.log().
How to Solve: you need to throttle the onPress function.
Example:
import React, { PureComponent } from 'react'
import { Text, View } from 'react-native'
import { throttle } from 'lodash'
export default class Test extends PureComponent {
constructor(props) {
super(props)
this.state = {
}
this.onPress = throttle(this.onPress, 500, {trailing: false})
}
onPress = () => {
console.log("going back")
this.props.navigation.pop();
//this.props.navigation.goBack();
}
render() {
return (
<View>
<Text>Hello World!</Text>
</View>
)
}
}
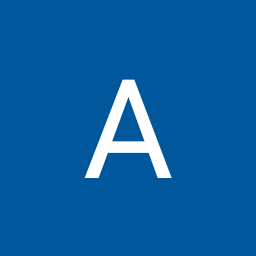
Comments
-
Ali almost 2 years
I have no idea what is causing this bug in my react native app. I'm using version 5 of the React Navigation library.
It randomly crashes the app sometimes. Google searching hasn't helped me understand what this is. It's very selective though which is a good(or a bad) thing.
So what does this mean and what could be causing it?
-
satya164 about 4 yearsIt can happen if you're trying to pop if there's no screens to go back to (e.g. click multiple times on the header back button). It's a development only error log. Not a crash. You can dismiss it and continue to use the app. The error won't be shown in production.
-
Ali about 4 yearsIn my case, the error was occurring while more data was being fetched from an API. This was on the initial screen so there was no header back button. Although, I was scrolling down on a few occasions.
-
Ali about 4 yearsYour explanation makes sense but the example doesn't apply to me. Could you think of any other examples?
-
satya164 about 4 yearsHard to say without looking at your code
-
Nikos about 4 yearsIt might be a bug: github.com/react-navigation/react-navigation/issues/6820
-
jave.web about 2 yearsmake sure, that if you call it from a function (like a callback) put
return
before :-) Also next time, please also add a code sample causing this or all code samples that could be causing this if you don't know :-)
-
-
Ali about 4 yearsThis is happening on the initial screen so I'm not executing such code.
-
Eric Hasegawa over 2 yearsNot sure why this is downvoted; this was perfect for my case! Thank you Aaron :)