Passing string to a function in C - with or without pointers?
Solution 1
The accepted convention of passing C-strings to functions is to use a pointer:
void function(char* name)
When the function modifies the string you should also pass in the length:
void function(char* name, size_t name_length)
Your first example:
char *functionname(char *name[256])
passes an array of pointers to strings which is not what you need at all.
Your second example:
char functionname(char name[256])
passes an array of chars. The size of the array here doesn't matter and the parameter will decay to a pointer anyway, so this is equivalent to:
char functionname(char *name)
See also this question for more details on array arguments in C.
Solution 2
Assuming that you meant to write
char *functionname(char *string[256])
Here you are declaring a function that takes an array of 256 pointers to char
as argument and returns a pointer to char. Here, on the other hand,
char functionname(char string[256])
You are declaring a function that takes an array of 256 char
s as argument and returns a char
.
In other words the first function takes an array of strings and returns a string, while the second takes a string and returns a character.
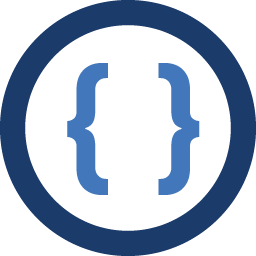
Admin
Updated on June 20, 2021Comments
-
Admin almost 3 years
When I'm passing a string to the function sometimes I use
char *functionname(char *name[256])
and sometimes I use it without pointers (for example:
char functionname(char name[256])
My question is,when do I need to use pointers ? Often I write programs without pointers and it works,but sometimes it doesn't.