Plotly Dash: How to change the button color when it is clicked?
To change the color of the button after it is clicked you need to update the corresponding style
property in the callback:
import dash
import dash_html_components as html
from dash.dependencies import Input, Output
app = dash.Dash(__name__)
white_button_style = {'background-color': 'white',
'color': 'black',
'height': '50px',
'width': '100px',
'margin-top': '50px',
'margin-left': '50px'}
red_button_style = {'background-color': 'red',
'color': 'white',
'height': '50px',
'width': '100px',
'margin-top': '50px',
'margin-left': '50px'}
app.layout = html.Div([
html.Button(id='button',
children=['click'],
n_clicks=0,
style=white_button_style
)
])
@app.callback(Output('button', 'style'), [Input('button', 'n_clicks')])
def change_button_style(n_clicks):
if n_clicks > 0:
return red_button_style
else:
return white_button_style
if __name__ == '__main__':
app.run_server(debug=True)
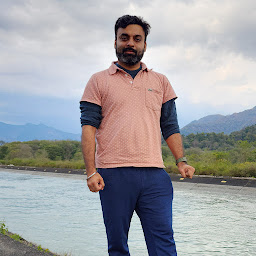
Amit Gupta
A full time learner, data science and Machine Learning. Getting better at python. Feel free to contact me: import pandas as pd print(''.join(pd.Series([109,111,99,pd.np.nan,46,108,105,97, 109,103,64,49,54,49,48,103,116,105,pd.np.nan, 109,97]).dropna().astype(int)[::-1].map(chr)))
Updated on June 05, 2022Comments
-
Amit Gupta almost 2 years
Hi I have 3 buttons designed in dash, I want to change the color of the button when it is clicked, I know there is to change in the @app.callback but I am not sure what to change. I want to keep everything same but the color should change to "Red"
Can anyone help me with this.
leftButtons = html.Div(id='framework-left-pane', children=[ dcc.Link( children = html.Button(leftButton1, id='leftbutton1', className='roundbutton', style={'backgroundColor': '#111100', 'color':'white','width':'100%', 'border':'1.5px black solid', 'height': '50px','text-align':'center', 'marginLeft': '20px', 'marginTop': 130}, n_clicks = 0)), dcc.Link( children = html.Button(leftButton2, id='leftbutton2', className='roundbutton', style={'backgroundColor': '#111100', 'color':'white','width':'100%' , 'border':'1.5px black solid','height': '50px','text-align':'center', 'marginLeft': '20px', 'marginTop': 20},n_clicks = 0)), dcc.Link( children = html.Button(leftButton3, id='leftbutton3', className='roundbutton', style={'backgroundColor': '#111100', 'color':'white','width':'100%', 'border':'1.5px black solid','height': '50px','text-align':'center', 'marginLeft': '20px', 'marginTop': 20},n_clicks = 0)), ],style={'width':'200px','position':'fixed'})
`
so right now with app.callback I am returning an image only
@app.callback( Output(component_id='tab_1_images', component_property='children'), [Input('leftbutton1', 'n_clicks'), Input('leftbutton2', 'n_clicks'), Input('leftbutton3', 'n_clicks')]) def update_output_div(n_clicks_A, n_clicks_B, n_clicks_C): return [a static image for each of the button]
-
Amit Gupta almost 4 yearsthere is not only 1 return, The color need to change but on clicking I need to display an image also, how to return both the values
-
Flavia Giammarino almost 4 yearsA given callback can return more than one output, see the section on "Multiple Outputs" in the Plotly documentation: dash.plotly.com/basic-callbacks.