Changing visibility of a Dash Component by updating other Component
Solution 1
You could place the Component you need to hide inside an html.div([])
and change its 'display' option to 'none' in a callback. The callback should have e.g a Dropdown as Input and the Component inside the html.div([])
as Output.
The following is a web app containing only a Dropdown and an Input Component that is visible/hidden based on the value of the Dropdown. It should work directly when copied:
import dash
from dash.dependencies import Input, Output
import dash_core_components as dcc
import dash_html_components as html
app = dash.Dash('example')
app.layout = html.Div([
dcc.Dropdown(
id = 'dropdown-to-show_or_hide-element',
options=[
{'label': 'Show element', 'value': 'on'},
{'label': 'Hide element', 'value': 'off'}
],
value = 'on'
),
# Create Div to place a conditionally visible element inside
html.Div([
# Create element to hide/show, in this case an 'Input Component'
dcc.Input(
id = 'element-to-hide',
placeholder = 'something',
value = 'Can you see me?',
)
], style= {'display': 'block'} # <-- This is the line that will be changed by the dropdown callback
)
])
@app.callback(
Output(component_id='element-to-hide', component_property='style'),
[Input(component_id='dropdown-to-show_or_hide-element', component_property='value')])
def show_hide_element(visibility_state):
if visibility_state == 'on':
return {'display': 'block'}
if visibility_state == 'off':
return {'display': 'none'}
if __name__ == '__main__':
app.run_server(debug=True)
Note that if multiple Components are placed inside the html.div([])
, the callback will still only change the 'display' property for the Component that it outputs. Thus, you could place other Dash Components inside the same Div without their visibility being affected.
Hope this answers your question properly.
Update (May 2020)
The Dash project and the User Documentation has evolved quite a bit since this answer was given two years ago. The docs now show multiple ways of accomplishing the sharing of state between callbacks, the first of which is by storing data in a hidden div as this answer suggests.
See the particular page in the docs here.
Solution 2
dcc.RadioItems(
id='show-table',
options=[{'label': i, 'value': i} for i in ['None', 'All', 'Alerts']],
value='None',
labelStyle={'display': 'inline-block'}
)
html.Div([
dash_table.DataTable(
id = 'datatable',
data=today_df.to_dict('records'),
columns=[{'id': c, 'name': c} for c in today_df.columns],
fixed_columns={ 'headers': True, 'data': 1 },
fixed_rows={ 'headers': True, 'data': 0 },
style_cell={
# all three widths are needed
'minWidth': '150px', 'width': '150px', 'maxWidth': '500px',
'whiteSpace': 'no-wrap',
'overflow': 'hidden',
'textOverflow': 'ellipsis',
},
style_table={'maxWidth': '1800px'},
filter_action="native",
sort_action="native",
sort_mode='multi',
row_deletable=True),
], style={'width': '100%'}, id='datatable-container')
@app.callback(
dash.dependencies.Output('datatable-container', 'style'),
[dash.dependencies.Input('show-table', 'value')])
def toggle_container(toggle_value):
print(toggle_value, flush=True)
if toggle_value == 'All':
return {'display': 'block'}
else:
return {'display': 'none'}
Related videos on Youtube
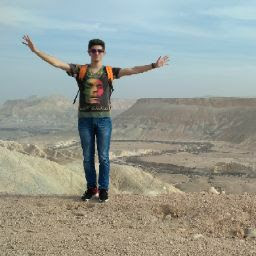
Иван Ситников
Updated on July 09, 2022Comments
-
Иван Ситников almost 2 years
I need to hide some Components, for example by clicking on a checkbox (for example, a graph or a table). However, the documentation did not provide a suitable section for this purpose. Thanks in advance!
-
Hoog almost 5 yearsWhile this code may answer the question, it would be better to explain how it solves the problem without introducing others and why to use it. Code-only answers are not useful in the long run.
-
DerDressing over 3 yearsIs it possible to hide the element by default? If I try in your code: style= {'display': 'none'} # <-- This is the line... the input component is not shown at all. Even if I switch the dropdown to 'on'.