populate a html list control using .NET
Solution 1
you can even use that HTML, adding runat="server" you will be able to treat it as a HTMLControl no mater what control it is, I do this often with div's
<ul id="myList" runat="server" class="myClass">
<li class="myItemClass">Item 1</li>
<li class="myItemClass">Item 2</li>
</ul>
then you get that HTMLControl and play with it
HtmlGenericControl li;
for (int x = 3; x <= 10; x++)
{
li = new HtmlGenericControl("li");
li.Attributes.Add("class", "myItemClass");
li.InnerText = "Item " + x;
myList.Controls.Add(li);
}
you will end up with:
<ul id="myList" runat="server" class="myClass">
<li class="myItemClass">Item 1</li>
<li class="myItemClass">Item 2</li>
<li class="myItemClass">Item 3</li>
<li class="myItemClass">Item 4</li>
<li class="myItemClass">Item 5</li>
<li class="myItemClass">Item 6</li>
<li class="myItemClass">Item 7</li>
<li class="myItemClass">Item 8</li>
<li class="myItemClass">Item 9</li>
<li class="myItemClass">Item 10</li>
</ul>
of course that you can use an ordered or unorderer list, they also below to the ASP.NET Web Controls.
<asp:BulletedList runat="server" ...
Solution 2
You could use asp:BulletedList like
<asp:BulletedList ID="MyList1" CssClass="MyClass" runat="server">
<asp:ListItem Text="Item1" class="MyClass" />
</asp:BulletedList>
Add add code like
ListItem item = new ListItem("Item2");
item.Attributes.Add("class", "MyClass");
MyList1.Items.Add(item);
Or if for some specific reason you need to use the ul tag then you can add a runat="server" to it. E.g.
<ul id="MyList2" class="MyClass" runat="server">
<li class="MyClass">Item1</li>
</ul>
With code like
HtmlGenericControl li = new HtmlGenericControl("li");
li.Attributes.Add("class", "MyClass");
li.InnerText = "Item2";
MyList2.Controls.Add(li);
Solution 3
The simplest way you can solve this problem is by using the asp repeater control
<ul id="myList" class='myClass'>
<asp:Repeater ID="repeaterMyList" Runat="server">
<ItemTemplate>
<li class="myItemClass">
<%# Eval("Item") %>
</li>
</ItemTemplate>
</asp:Repeater>
</ul>
[Edit] - Do remember to set the datasource on repeaterMyList and call databind on the repeater control in the codebehind.
repeaterMyList.DataSource = someDataTable;
repeaterMyList.DataBind();
Solution 4
I'm assuming that there is a valid reason for you not to use the BulletedList webserver control. Anyway, this is an interesting programming exercise that illustrates the internals of the Htmlservercontrol architecture and how they map to simple HTML tags.
The HTML ul
and li
tags are not directly mapped as HTMLServercontrols. This means that even if you add a runat="server"
attribute to the list, it's contents will not be directly accessible as listitems.
However, all controls not directly mapped as Html server controls are accessible via the HtmlGenericControl
class. This makes it possible to create and modify such controls dynamically.
The solution, therefore, is twofold:
- Make the unordered list
runat="server"
so that you can access it in server-side code. Also, you should make the existing items in the listrunat="server"
, else they will be only be accessible as anLiteralControl
that contains the first two listitems as plain text. - In code, access the contents of the list and add a new HtmlGenericControl of type "li" to it.
The following (bare-bones simple) page demonstrates this procedure :
<%@ Page Language="VB" AutoEventWireup="false" %>
<%@ Import Namespace="System.Collections.Generic" %>
<script runat="server">
Private Shared addedItems As List(Of HtmlGenericControl)
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
If Not Page.IsPostBack Then
'On first load, instantiate the List.
addedItems = New List(Of HtmlGenericControl)
End If
End Sub
Protected Sub btn1_Click(ByVal sender As Object, ByVal e As System.EventArgs)
'Add the previously created items to the UL list.
'This step is necessary because
'...the previously added items are lost on postback.
For i As Integer = 0 To addedItems.Count - 1
myList.Controls.Add(addedItems.Item(i))
Next
'Get the existing no. of items in the list
Dim count As Integer = myList.Controls.Count
'Create a new list item based on input in textbox.
Dim li As HtmlGenericControl = CreateBulletItem()
'Add the new list item at the end of the BulletedList.
myList.Controls.AddAt(count, li)
'Also add this newly created list item to the generic list.
addedItems.Add(li)
End Sub
Private Function CreateBulletItem() As HtmlGenericControl
Dim li As New HtmlGenericControl("li")
li.InnerText = txtNewItem.Value
li.Attributes("class") = "myItemClass"
Return li
End Function
</script>
<html>
<head runat="server">
<title>Test Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<ul id="myList" class='myClass' runat="server">
<li runat="server" class="myItemClass">Item 1</li>
<li runat="server" class="myItemClass">Item 2</li>
</ul>
<input type="text" id="txtNewItem" runat="server" />
<asp:Button ID="btn1" runat="server" Text="Add" OnClick="btn1_Click" />
</div>
</form>
</body>
</html>
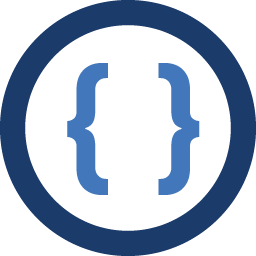
Admin
Updated on August 11, 2022Comments
-
Admin almost 2 years
I have a list defined like so:
<ul id="myList" class='myClass'> <li class="myItemClass">Item 1</li> <li class="myItemClass">Item 2</li> </ul>
using .NET how can I add items to the list dynamically? I also need to specify the class name on each new item