Post array of strings to web API method
Solution 1
For passing simply types, the data to post must take the form of a name value pair with the name portion being an empty string. So you need to make the Ajax call like so:
$.ajax({
type: "POST",
url: "/api/values",
data: { "": list },
dataType: "json",
success: function() { alert("it worked!"); },
failure: function() { alert("not working..."); }
});
Additionally, on your Web API action, annotate it w/ the [FromBody] attribute. Something like:
public void Post([FromBody]string[] values)
That should do the trick.
Solution 2
You should pass the list
itself, and not any other object wrapping it.
E.g. pass the following:
var list = ["a", "b", "c", "d"];
in
$.ajax({
type: "POST",
url: "/api/scheduledItemPriceStatus/updateStatusToDelete",
// Pass the list itself
data: list,
dataType: "json",
traditional: true,
success: function() { alert("it worked!"); },
failure: function() { alert("not working..."); }
});
Your method signature on server is correct.
Solution 3
use var jsonText = { data: JSON.stringify(list)}
Solution 4
In the backend, you could use FormDataCollection.GetValues(string key)
to return an array of strings for that parameter.
public HttpResponseMessage UpdateStatusToDelete(FormDataCollection formData) {
string[] data = formData.GetValues("data");
...
}
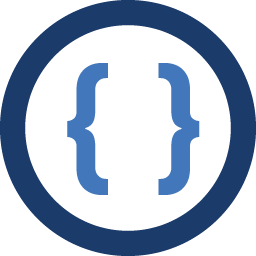
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
this is my client side ajax call:
var list = ["a", "b", "c", "d"]; var jsonText = { data: list }; $.ajax({ type: "POST", url: "/api/scheduledItemPriceStatus/updateStatusToDelete", data: jsonText, dataType: "json", traditional: true, success: function() { alert("it worked!"); }, failure: function() { alert("not working..."); } });
this is chrome network header:
Request URL:http://localhost:2538/api/scheduledItemPriceStatus/updateStatusToDelete Request Method:POST Request Headersview source Accept:application/json, text/javascript, */*; q=0.01 Accept-Charset:ISO-8859-1,utf-8;q=0.7,*;q=0.3 Accept-Encoding:gzip,deflate,sdch Accept-Language:en-US,en;q=0.8 Connection:keep-alive Content-Length:27 Content-Type:application/x-www-form-urlencoded; charset=UTF-8 Host:localhost:2538 Origin:http://localhost:2538 Referer:http://localhost:2538/Pricing/ScheduledItemPrices User-Agent:Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.11 (KHTML, like Gecko) Chrome/23.0.1271.97 Safari/537.11 X-Requested-With:XMLHttpRequest
Form Dataview URL encoded
data:a data:b data:c data:d
this is my webapi controller method:
public HttpResponseMessage UpdateStatusToDelete(string[] data)
result:
when I debug, the data parameter in UpdateStatusToDelete returns
{string[0]}
instead of data:a data:b data:c data:dWhat am I doing wrong? Any help is really appreciated.
-
MethodMan over 11 yearsChris there is now from what I can tell that the list portion will compile.. check the code out and tell me.... [] should be { }
-
Shaswat Rungta over 7 yearsI still get a null values for values using this approach. the one different is My API also has Non body parameters. Something like public void Post( string id, [FromBody]string[] values)