Prevent form submission on Enter key press
Solution 1
if(characterCode == 13)
{
return false; // returning false will prevent the event from bubbling up.
}
else
{
return true;
}
Ok, so imagine you have the following textbox in a form:
<input id="scriptBox" type="text" onkeypress="return runScript(event)" />
In order to run some "user defined" script from this text box when the enter key is pressed, and not have it submit the form, here is some sample code. Please note that this function doesn't do any error checking and most likely will only work in IE. To do this right you need a more robust solution, but you will get the general idea.
function runScript(e) {
//See notes about 'which' and 'key'
if (e.keyCode == 13) {
var tb = document.getElementById("scriptBox");
eval(tb.value);
return false;
}
}
returning the value of the function will alert the event handler not to bubble the event any further, and will prevent the keypress event from being handled further.
NOTE:
It's been pointed out that keyCode
is now deprecated. The next best alternative which
has also been deprecated.
Unfortunately the favored standard key
, which is widely supported by modern browsers, has some dodgy behavior in IE and Edge. Anything older than IE11 would still need a polyfill.
Furthermore, while the deprecated warning is quite ominous about keyCode
and which
, removing those would represent a massive breaking change to untold numbers of legacy websites. For that reason, it is unlikely they are going anywhere anytime soon.
Solution 2
Use both event.which
and event.keyCode
:
function (event) {
if (event.which == 13 || event.keyCode == 13) {
//code to execute here
return false;
}
return true;
};
Solution 3
event.key === "Enter"
More recent and much cleaner: use event.key
. No more arbitrary number codes!
NOTE: The old properties (
.keyCode
and.which
) are Deprecated.
const node = document.getElementsByClassName("mySelect")[0];
node.addEventListener("keydown", function(event) {
if (event.key === "Enter") {
event.preventDefault();
// Do more work
}
});
Modern style, with lambda and destructuring
node.addEventListener("keydown", ({key}) => {
if (key === "Enter") // Handle press
})
Solution 4
If you're using jQuery:
$('input[type=text]').on('keydown', function(e) {
if (e.which == 13) {
e.preventDefault();
}
});
Solution 5
Detect Enter key pressed on whole document:
$(document).keypress(function (e) {
if (e.which == 13) {
alert('enter key is pressed');
}
});
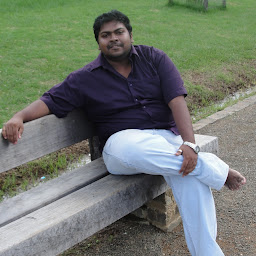
Dushan Perera
❤️ building super 🚅 fast 🏎️ web experience 🚀 Azure functions <⚡> is my recent area of interest. Former ASP.NET MVP Please feel free to suggest improvements to my posts
Updated on January 01, 2022Comments
-
Dushan Perera over 2 years
I have a
form
with two text boxes, one select drop down and one radio button. When the enter key is pressed, I want to call my JavaScript function, but when I press it, theform
is submitted.How do I prevent the
form
from being submitted when the enter key is pressed? -
Dushan Perera almost 15 yearsI cant override onsubmit as i have another form submit in this page
-
the_drow almost 15 yearsyes you can. Javascript is prototype based language. document.forms["form_name"].onsubmit = fucntion() {}
-
Dushan Perera almost 15 yearsI have another delete procedure to run when the form is submitted.So i want to maintain as it is
-
Admin over 12 yearsIs this a situation where PreventDefualt can be used?
-
Les about 12 yearsYes, instead of the return false.
-
Wk_of_Angmar about 11 yearsInstead of eval, you could build an array of the valid functions, then use .indexOf() to check whether the user's input is in that array, and call the function if it is. Reduces scope for errors/users screwing up.
-
Alex Spurling about 11 yearsWhy both event.which and event.keyCode?
-
user568109 almost 11 yearsSome browsers support
which
others supportkeyCode
. It is good practice to include both. -
Manish Singh over 10 yearsAlso use the
keydown
event instead ofkeyup
orkeypress
-
Pierre-Olivier Vares over 9 yearskeyup is fired AFTER the submission, and can't therefore cancel it.
-
Don Cheadle over 8 years@Agiagnoc but what event should this be attached to ?
-
john-jones over 8 years@manish, keypress gives you more information to play with than keydown/up. stackoverflow.com/a/9905293/322537
-
Anders Kitson almost 8 yearsIE does not support just
event.keyCode
, tested on a new windows tablet and return key wasn't firing my functions. Addingwhich
as stated above should fix any IE bugs. -
Donatas Navidonskis over 6 yearsset var charCode = parseInt((e.which) ? e.which : e.keyCode); and then compare if(charCode == 13) { // execute } return false;
-
John Slegers over 6 years
-
eddyparkinson about 6 yearsWhy has client_id etc been included here? Is the code legit?
-
Nico Haase about 6 yearsThis question is more than eight years old. Typescript did not exist then. So are you sure you're not answering an other question?
-
clockw0rk over 5 yearsisn't this just a macro or alias? is there any benefit in terms of performance by using this code?
-
Gibolt over 5 years
keyCode
is deprecated. This is more readable and maintainable than a magic integer in your code -
Jing He almost 4 years@Shyju if you want to run both: the action defined in the form tag and also the javascript triggered when pressing the submit button, you can just remove the code
return false;
, then both action will be triggered. -
Danziger over 3 yearsWhile I agree with your note about
keyCode
andwhich
being deprecated, I think developers should help make that transition by not supporting such legacy browsers, especially now that IE is officially dead and Edge has adopted Chromium. Anyway, a nice tool to inspect the different identifiers keys have is keyjs.dev -
Danziger over 3 yearsFor those wondering how to find the
key
value for different keys, you can use keyjs.dev -
Alexander Nied about 3 yearsIn 2021, I believe this is the correct answer.
-
Tamil Vendhan Kanagarasu over 2 yearsReadable, yes! Cleaner? 13 is more clean than
Enter
. There was no questions like, is it "Enter" or "enter". If you can remember "Enter", you can always remember13
. It takes less room. -
Greggory Wiley about 2 yearsOnly works if you install jquery.
-
Dozie about 2 years@Josh, @JohnSlegers, @Danziger KeyboardEvent.code is now supported in major browser versions. So this response can be refactored to use:
if (e.code === "Enter")
-
NetXpert almost 2 years"abitrary"?! It has existed as a standard since the development of ASCII, ~1961! Itself having been based-upon / derived-from work that began with teletype machines starting 40 years before that! Though it may have begun as such, here, now, in 2022, 60+ years on from the development of that standard, and the ubiquitous implementations that stem therefrom, knowing that 0x0d / (dec)13 represents a carriage-return is well-beyond being just some 'arbitrary' value, and anyone who's been coding for longer than a year should know it as naturally/intrinsically as they know how to breathe air...🙄
-
Gibolt almost 2 yearsYes, the history is real. However the numbers are arbitrary, just like the arrangement of keys on a keyboard. Few devs have the list of char/key mappings memorized