Printing a new line in assembly language with MS-DOS int 21h system calls
Solution 1
Code for printing new line
MOV dl, 10
MOV ah, 02h
INT 21h
MOV dl, 13
MOV ah, 02h
INT 21h
ascii ---> 10 New Line
ascii ---> 13 Carriage Return
That is code in assembly for new line, code is inspirated with writing machine. Our professor told us the story but I'm not good at english.
Cheers :)
Solution 2
Well, first off:
mov DL, 0DH
mov DL, 0AH
int 21H
Isn't going to do you any good. You load 0Dh into DL and then immediately overwrite it with 0Ah without ever having used the first value... You need to make your call (int 21h) on BOTH characters...
Furthermore, you're using DL for newlines overwrites the prior use for the character... You need to save and restore that value as necessary.
Solution 3
100% works.
CR equ 0DH
LF equ 0AH
main:
mov DL, 'A'
while1:
mov AH,02H ;print character
int 21H
mov BL, DL ;store the value of DL before using DL for print new line
mov DL, 10 ;printing new line
mov AH, 02h
int 21h
mov DL, 13
mov AH, 02h
int 21h
mov DL, BL ;return the value to DL
cmp DL, 'Z'
je exit
add DL, 1 ;store in DL the next character
jmp while1
exit:
mov AH,4CH
int 21h
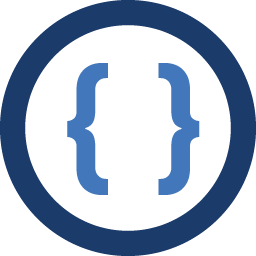
Admin
Updated on May 20, 2020Comments
-
Admin almost 4 years
I've been trying to print a new line while also printing the alphabet using assembly language in nasmide for the past few days and can't get it, what I've tried so far has either printed nothing, printed just A or printed a multitude of symbols, Google hasn't been helpful to me so I decided to post here.
My code so far is
CR equ 0DH LF equ 0AH main: mov AH,02H mov CX,26 mov DL, 'A' while1: cmp DL, 'A' add DL, 01H int 21H mov DL, 0DH mov DL, 0AH int 21H cmp DL, 'Z' je Next jmp while1 Next: mov AH,4CH int 21h