Put 2 columns of recycler view every row
11,069
Replace your LinearLayoutManager
for a GridLayoutManager
like this:
private GridLayoutManager mGridLayoutManager;
// ...
mGridLayoutManager = new GridLayoutManager(getActivity(), 2);
Then you can use it the same way as the LinearLayoutManager
.
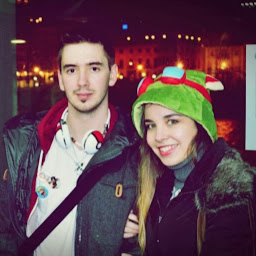
Author by
Juanfri Lira
Updated on June 04, 2022Comments
-
Juanfri Lira almost 2 years
I would like to put 2 columns of recyclerview every row.
Right now my design is like this:
and i would like to put 2 so the scroll is not to long.
I have this view inside a fragment and I use a recyclerview adapter but I have no clue how to tell it to use its parent with, so it can fill the width of the screen. In the fragment I recive a JSON from an online database using an AsyncTask and fill in the recyclerview on the onPostExecute of the AsyncTask.
If anyone has any Idea how i can do it, I can't seem to find this anywhere
Thx!
My recyclerview Adapter:
package com.example.juanfri.seguridadmainactivity; /** * Created by jlira on 30/05/2017. */ import android.support.v7.widget.RecyclerView; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ImageView; import android.widget.TextView; import com.squareup.picasso.Picasso; import java.util.ArrayList; /** * Created by Juanfri on 29/05/2017. */ public class RecyclerAdapterSerie extends RecyclerView.Adapter<RecyclerAdapterSerie.SerieHolder> { private ArrayList<Serie> mSerie; @Override public RecyclerAdapterSerie.SerieHolder onCreateViewHolder(ViewGroup viewGroup, int i) { View inflatedView = LayoutInflater.from(viewGroup.getContext()) .inflate(R.layout.recyclerview_card, viewGroup, false); return new SerieHolder(inflatedView); } @Override public void onBindViewHolder(RecyclerAdapterSerie.SerieHolder holder, int i) { Serie itemPhoto = mSerie.get(i); holder.bindPhoto(itemPhoto); } @Override public int getItemCount() { return mSerie.size(); } public RecyclerAdapterSerie(ArrayList<Serie> serie) { mSerie = serie; } public static class SerieHolder extends RecyclerView.ViewHolder implements View.OnClickListener { //2 private ImageView mItemImage; private TextView mItemDate; private TextView mItemDescription; private Serie serie; //3 private static final String PHOTO_KEY = "PHOTO"; //4 public SerieHolder(View v) { super(v); mItemImage = (ImageView) v.findViewById(R.id.item_image); mItemDate = (TextView) v.findViewById(R.id.item_date); mItemDescription = (TextView) v.findViewById(R.id.item_description); v.setOnClickListener(this); } //5 @Override public void onClick(View v) { /*Context context = itemView.getContext(); Intent showPhotoIntent = new Intent(context, Pelicula.class); showPhotoIntent.putExtra(PHOTO_KEY, peli); context.startActivity(showPhotoIntent);*/ } public void bindPhoto(Serie mserie) { serie = mserie; String Nombre = mserie.getNombreSerie(); if(Nombre.length() >= 25) { Nombre = Nombre.substring(0,22); Nombre = Nombre + "..."; } Picasso.with(mItemImage.getContext()).load(mserie.getPoster()).into(mItemImage); mItemDate.setText(Nombre); mItemDescription.setText(Integer.toString(mserie.getIdTMDB())); } } }
My fragment (where i create the recyclerview)
package com.example.juanfri.seguridadmainactivity; import android.app.ProgressDialog; import android.os.AsyncTask; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v4.app.Fragment; import android.support.v7.widget.LinearLayoutManager; import android.support.v7.widget.RecyclerView; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.Button; import android.widget.Toast; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import java.util.ArrayList; import static android.content.ContentValues.TAG; /** * Created by jlira on 06/06/2017. */ public class FragmentoSeriesPelis extends Fragment { public final String API = "5e2780b2117b40f9e4dfb96572a7bc4d"; public final String URLFOTO ="https://image.tmdb.org/t/p/original/"; private ProgressDialog pDialog; private RecyclerView recyclerView; private LinearLayoutManager mLinearLayoutManager; private ArrayList<Serie> series; private ArrayList<Pelicula> Pelis; private RecyclerAdapterSerie mAdapterSerie; private RecyclerAdapterPelicula mAdapterPeli; private int pagina; private String url; private Button CargarMas; private String tipo; private int MaxPag; @Nullable @Override public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { View view = inflater.inflate(R.layout.series_pelis_layout, container, false); view.setTag(TAG); Bundle args = getArguments(); series = new ArrayList<>(); Pelis = new ArrayList<>(); MaxPag = 2; pagina = args.getInt("Page"); url = args.getString("url"); tipo = args.getString("Tipo"); recyclerView = (RecyclerView) view.findViewById(R.id.recyclerViewSerieshoy); //mLinearLayoutManagerSerie = new LinearLayoutManager(getActivity(), LinearLayoutManager.HORIZONTAL, false); //recyclerViewSerie.setLayoutManager(mLinearLayoutManagerSerie); //mAdapterSerie = new RecyclerAdapterSerie(seriesHoy); //recyclerViewSerie.setAdapter(mAdapterSerie); new GetSeriesHoy().execute(); CargarMas = (Button) view.findViewById(R.id.buttonCargarMas); CargarMas.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if(pagina+1<=MaxPag) { pagina++; new GetSeriesHoy().execute(); } else { Toast.makeText(getActivity(), "No hay mas resultados", Toast.LENGTH_LONG).show(); } } }); return view; } @Override public void onViewCreated(View view, @Nullable Bundle savedInstanceState) { super.onViewCreated(view, savedInstanceState); getActivity().setTitle("Series de Hoy"); } private class GetSeriesHoy extends AsyncTask<Void, Void, Void> { @Override protected void onPreExecute() { super.onPreExecute(); // Showing progress dialog pDialog = new ProgressDialog(getActivity()); pDialog.setMessage("Please wait..."); pDialog.setCancelable(false); pDialog.show(); } @Override protected Void doInBackground(Void... arg0) { HttpHandler sh = new HttpHandler(); // Making a request to url and getting response //url = "https://api.themoviedb.org/3/tv/airing_today?api_key="+API+"&language=en-US&page="+pagina; String aux = url+pagina; String jsonStr = sh.makeServiceCall(aux); if (jsonStr != null) { try { JSONObject jsonObj = new JSONObject(jsonStr); // Getting JSON Array node MaxPag = jsonObj.getInt("total_pages"); JSONArray contacts = jsonObj.getJSONArray("results"); // looping through All Contacts for (int i = 0; i < contacts.length(); i++) { JSONObject c = contacts.getJSONObject(i); int IdSerie = i; int idTMDB = c.getInt("id"); String nombreSerie; String poster =URLFOTO + c.getString("poster_path"); if(tipo.equalsIgnoreCase("Series")) { nombreSerie = c.getString("name"); Serie nuevo = new Serie(); nuevo.setIdSerie(IdSerie); nuevo.setNombreSerie(nombreSerie); nuevo.setIdTMDB(idTMDB); nuevo.setPoster(poster); series.add(nuevo); } else { nombreSerie = c.getString("title"); Pelicula nuevo = new Pelicula(); nuevo.setIdPelicula(IdSerie); nuevo.setNombrePelicula(nombreSerie); nuevo.setIdTMDB(idTMDB); nuevo.setPoster(poster); Pelis.add(nuevo); } } } catch (final JSONException e) { Toast.makeText(getActivity(), "Json parsing error: " + e.getMessage(), Toast.LENGTH_LONG) .show(); } } else { Toast.makeText(getActivity(), "Couldn't get json from server. Check LogCat for possible errors!", Toast.LENGTH_LONG) .show(); } return null; } @Override protected void onPostExecute(Void result) { super.onPostExecute(result); // Dismiss the progress dialog if (pDialog.isShowing()) pDialog.dismiss(); /** * Updating parsed JSON data into ListView * */ //Aqui Realizar la RecycleView BuildUp if(tipo.equalsIgnoreCase("Series")) { mLinearLayoutManager = new LinearLayoutManager(getActivity(), LinearLayoutManager.VERTICAL, false); recyclerView.setLayoutManager(mLinearLayoutManager); mAdapterSerie = new RecyclerAdapterSerie(series); recyclerView.setAdapter(mAdapterSerie); }else { mLinearLayoutManager = new LinearLayoutManager(getActivity(), LinearLayoutManager.VERTICAL, false); recyclerView.setLayoutManager(mLinearLayoutManager); mAdapterPeli = new RecyclerAdapterPelicula(Pelis); recyclerView.setAdapter(mAdapterPeli); } } } }