Pygame: Unable to open .mp3 file
Solution 1
There's another mixer method, music
, that you should probably be using - music
supports mp3, but sound
doesn't. Try this.
pygame.mixer.music.load('JUST DO IT.mp3')
pygame.mixer.music.play()
time.sleep(2)
pygame.mixer.music.stop()
^that's a pretty dank meme by the way
Solution 2
So pygame only allows for files that are either OGG or a compressed WAV file to be played using Sound. However you can use music instead of sound to play mp3 files. Plus next time you can look up the info on the PyGame documentation here. https://www.pygame.org/docs/
import pygame, sys, time
from pygame.locals import *
pygame.init()
DISPLAYSURF = pygame.display.set_mode((400, 300))
pygame.display.set_caption('Memes.')
pygame.mixer.music.load("foo.mp3")
pygame.mixer.music.play()
time.sleep(2)
pygame.mixer.music.stop()
while True: # Main Loop
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
pygame.display.update()
just replace the foo.mp3 with your file
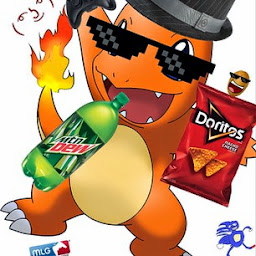
Yolo McSwaggins
Updated on July 09, 2022Comments
-
Yolo McSwaggins almost 2 years
So... Whenever I try to run this block of code:
import pygame, sys, time from pygame.locals import * pygame.init() DISPLAYSURF = pygame.display.set_mode((400, 300)) pygame.display.set_caption('Memes.') meme = pygame.mixer.Sound('JUST DO IT.mp3') meme.play() time.sleep(2) meme.stop() while True: # Main Loop for event in pygame.event.get(): if event.type == QUIT: pygame.quit() sys.exit() pygame.display.update()
I get this error:
Traceback (most recent call last): File "C:\Users\Slay-Slay\Desktop\Python\Python Code\play soun.py", line 9, in <module> meme = pygame.mixer.Sound('JUST DO IT.mp3') pygame.error: Unable to open file 'JUST DO IT.mp3'
Both the code and the sound are in the same exact folder, before you ask. I don't know what the problem could be... I thought pygame supported .mp3?
EDIT: I just tried it with the pygame.mixer.music.load() function... And that didn't work either. It instead gave me this error:
Traceback (most recent call last): File "C:\Users\Slay-Slay\Desktop\Python\Python Code\play soun.py", line 9, in <module> pygame.mixer.music.load('JUST DO IT.mp3') pygame.error: Couldn't read from 'JUST DO IT.mp3'
I also tried different formats, such as WAV or OGG. Neither worked. I tried all 3 formats on the 2 different functions. All returned the same errors. "Couldn't read from" for music.load, and "Unable to open file" for Sound.