pyshark - data from TCP packet
Solution 1
If you are using a .pcap file, once you have read the file using
cap = pyshark.FileCapture('vox.pcap')
and say, you want to read the data of the 2nd packet, and you are sure such a field exists, try:
pkt = cap[1]
print pkt.tcp.data
To see the options available for pkt.tcp, use:
dir(pkt.tcp)
It will return all the available options for pkt.tcp
Solution 2
Using pyshark 0.3.7.11 with python 3.6 and Assuming you have loaded a capture file, you can get the tcp payload with:
p = capfile[0] # or any packet you know has a tcp layer
payload = p.tcp.payload
p.tcp.payload Out[139]: '6f:00:2c:00:01:00:02:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:00:02:00:00:00:00:00:b2:00:1c:00:81:00:00:00:01:00:03:00:03:00:02:00:00:00:00:00:64:00:01:00:04:00:02:00:6b:00:03:00
You can find out the fields you can access with the following:
dir(p) # for entire packet
dir(p.ip) # for ip layer
dir(p.tcp) # for tcp layer
Print out information in a nice pretty format:
print(p.tcp) # one way
p.tcp.pretty_print() # using pretty print
Not sure what else might be useful to you. Docs are at https://github.com/KimiNewt/pyshark
Solution 3
you could do:
import pyshark
import sys
cap = pyshark.FileCapture(sys.argv[-1])
for i in cap:
try:
print(i.data.data)
except:
print("no data")
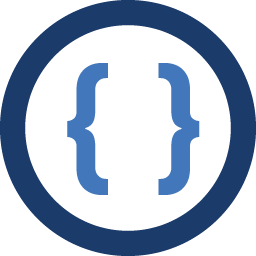
Admin
Updated on July 26, 2022Comments
-
Admin almost 2 years
Is there anyway to get the payload of a TCP packet using pyshark?
I am trying to compare the data sections of different packets across multiple TCP streams but I can't find a way to get at the data of the packet.
pkt['tcp'].data
does not seem to exist.