Python cannot handle numbers string starting with 0. Why?
Solution 1
My guess is that since 012
is no longer an octal literal constant in python3.x, they disallowed the 012
syntax to avoid strange backward compatibility bugs. Consider your python2.x script which using octal literal constants:
a = 012 + 013
Then you port it to python 3 and it still works -- It just gives you a = 25
instead of a = 21
as you expected previously (decimal). Have fun tracking down that bug.
Solution 2
From the Python 3 release notes http://docs.python.org/3.0/whatsnew/3.0.html#integers
Octal literals are no longer of the form
0720
; use0o720
instead.
The 'leading zero' syntax for octal literals in Python 2.x was a common gotcha:
Python 2.7.3
>>> 010
8
In Python 3.x it's a syntax error, as you've discovered:
Python 3.3.0
>>> 010
File "<stdin>", line 1
010
^
SyntaxError: invalid token
You can still convert from strings with leading zeros same as ever:
>>> int("010")
10
Related videos on Youtube
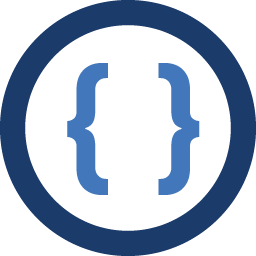
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
I just executed the following program on my python interpreter:
>>> def mylife(x): ... if x>0: ... print(x) ... else: ... print(-x) ... >>> mylife(01) File "<stdin>", line 1 mylife(01) ^ SyntaxError: invalid token >>> mylife(1) 1 >>> mylife(-1) 1 >>> mylife(0) 0
Now, I have seen this but as the link says, the 0 for octal does not work any more in python (i.e. does not work in python3). But does that not mean that the the behaviour for numbers starting with 0 should be interpreted properly? Either in base-2 or in normal base-10 representation? Since it is not so, why does python behave like that? Is it an implementation issue? Or is it a semantic issue?
-
Admin over 11 yearsYup. Seems like a good explanation, as possible design decision. May be after two years, when most of the stuff has been ported, then, I think, the feature should be allowed again. Thanks!
-
Jon Clements over 11 yearsReminds of the "Why do programmers confuse Christmas and Halloween" - "Because dec(25) is oct(31)" ;P
-
mgilson over 11 years@JonClements -- I'd never seen that one before -- Quite clever -- I suppose that was probably mentioned in one of the CS classes I never took.
-
Jean-François Fabre over 6 years@mgilson probably the good reason. Which explains why
000
is still valid: stackoverflow.com/questions/43071916/…