Python: Invalid Syntax Using Global Variable
Solution 1
You are putting global
where it's unneeded:
global check = 1
You don't need global
here, check
is already global
here.
if(global check == 1)
, global check = global check + 1
is also not a valid use of global
.
Instead, declare check
as global
in main():
def main():
global check
Solution 2
Use like this
check=10
def function() :
global check
if(check==1):
#blablabla
The global keyword helps you to bring value to your function... Then it's useless and unnecessary to use that in your function again...
For simplicity, global helps you to bring your friend to your home, and then there is no need for you to bring him again since he is already here...
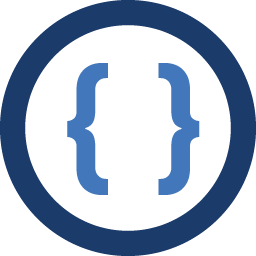
Admin
Updated on July 16, 2020Comments
-
Admin almost 4 years
First off, i have to tell you that i am completely new to coding, so the problem i have might be caused by the most stupid misstake ever, and if so, im sorry!
I am trying to make a calculator which is able to calculate +,-,*,/. It's also supposed to give an error message and ask for a new operation symbol if the one recieved was invalid. To let the computer know if the funtion "main" is being run because it recieved an invalid funtion, or if it is first time its being run, i am trying to use a global variable called "check". At the start, check is set to 1, and so the computer will use the first phrase when asking for an operation. If an invalid operation is entered, the variable "check" is incresed by one, which will lead to the second phrase (the error message) when it asks for a new operation.
The problem is that when i try to run the script, i get a syntax error on the first line, where "global check = 1". What am i doing wrong?
Below is my code:
global check = 1 #returns num1 + num2 def add(num1,num2): return num1 + num2 #returns num1 - num2 def sub(num1,num2): return num1 - num2 #returns num1 * num2 def mul (num1,num2): return num1 * num2 #returns num1 / num2 def div (num1,num2): return num1 / num2 #Main Function def main(): if(global check == 1): #checks if "main" has been read before, if it has, then it is read agian because of invalid operation, and the global "check" should be higher than 1. operation = input("Choose an operation! (+,-,*,/") else: operation = input("You must choose a valid operation! (+,-,*,/") if(operation != "+" and operation != "-" and operation != "*" and operation != "/"): global check = global check + 1 main() else: var1 = int(input("Enter number 1 :")) var2 = int(input("Enter number 2 :")) if(operation == "+"): print(add(var1,var2)) elif(operation == "-"): print(sub(var1,var2)) elif(operation == "*"): print(mul(var1,var2)) else: print(div(var1,var2)) main()