name 'times' is used prior to global declaration - But IT IS declared!
Solution 1
The global declaration is when you declare that times
is global
def timeit():
global times # <- global declaration
# ...
If a variable is declared global
, it can't be used before the declaration.
In this case, I don't think you need the declaration at all, because you're not assigning to times
, just modifying it.
Solution 2
From the Python documentation:
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
https://docs.python.org/reference/simple_stmts.html#global
So moving global times
to the top of the function should fix it.
But, you should try not to use global
in this situation. Consider using a class.
Solution 3
From the Python Docs
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
Solution 4
This program should work but may not work exactly as you intended. Please take note of the changes.
import time
times = []
def timeit():
input("Press ENTER to start: ")
start_time = time.time()
input("Press ENTER to stop: ")
end_time = time.time()
the_time = round(end_time - start_time, 2)
print(str(the_time))
times.append(the_time)
def main():
while True:
print ("Do you want to...")
print ("1. Time your solving")
print ("2. See your solvings")
dothis = input(":: ")
if dothis == "1":
timeit()
elif dothis == "2":
sorted_times = sorted(times)
sorted_times.reverse()
for curr_time in sorted_times:
print("%d - %f" % ((sorted_times.index(curr_time)+1), curr_time))
break
else:
print ("WTF? Please enter a valid number...")
main()
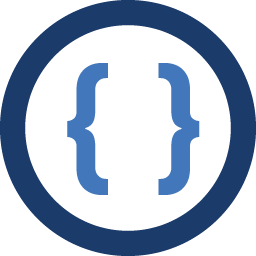
Admin
Updated on June 28, 2021Comments
-
Admin almost 3 years
I'm coding a small program to time and show, in a ordered fashion, my Rubik's cube solvings. But Python (3) keeps bothering me about times being used prior to global declaration. But what's strange is that IT IS declared, right on the beggining, as
times = []
(yes, it's a list) and then again, on the function (that's where he complains) astimes = [some, weird, list]
and "globaling" it withglobal times
. Here is my code, so you may analyse it as you want:import time times = [] def timeit(): input("Press ENTER to start: ") start_time = time.time() input("Press ENTER to stop: ") end_time = time.time() the_time = round(end_time - start_time, 2) print(str(the_time)) times.append(the_time) global times main() def main(): print ("Do you want to...") print ("1. Time your solving") print ("2. See your solvings") dothis = input(":: ") if dothis == "1": timeit() elif dothis == "2": sorte_times = times.sort() sorted_times = sorte_times.reverse() for curr_time in sorted_times: print("%d - %f" % ((sorted_times.index(curr_time)+1), curr_time)) else: print ("WTF? Please enter a valid number...") main() main()
Any help would be very appreciated as I'm new in the world of Python :)
-
Admin over 14 yearsDarn it! Now, when I choose option 2 to show me my results, this shows up: AttributeError: 'NoneType' object has no attribute 'reverse', referring to sorted_times = sorte_times.reverse()
-
John Millikin over 14 yearsThat's because
times.sort()
returnsNone
. You should use eithertimes.sort(); print times
orprint sorted(times)
. -
Sven Eberth almost 3 yearsWhile this code may answer the question, providing additional context regarding how and/or why it solves the problem would improve the answer's long-term value.
-
thePythonNoob almost 3 yearsI already told. Because you must write the "global<varname>" at start
-
thePythonNoob almost 3 yearsthis was the problem