Python: Converting string into decimal number
201,972
Solution 1
If you want the result as the nearest binary floating point number use float
:
result = [float(x.strip(' "')) for x in A1]
If you want the result stored exactly use Decimal
instead of float
:
from decimal import Decimal
result = [Decimal(x.strip(' "')) for x in A1]
Solution 2
If you are converting price (in string) to decimal price then....
from decimal import Decimal
price = "14000,45"
price_in_decimal = Decimal(price.replace(',','.'))
No need for the replace if your strings already use dots as a decimal separator
Solution 3
You will need to use strip()
because of the extra bits in the strings.
A2 = [float(x.strip('"')) for x in A1]
Solution 4
use the built in float() function in a list comprehension.
A2 = [float(v.replace('"','').strip()) for v in A1]
Solution 5
A2 = [float(x.strip('"')) for x in A1]
works, @Jake , but there are unnecessary 0s
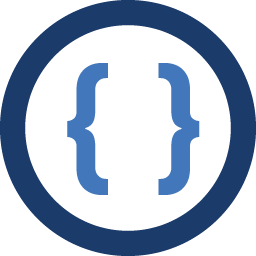
Author by
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
I have a python list with strings in this format:
A1 = [' "29.0" ',' "65.2" ',' "75.2" ']
How do I convert those strings into decimal numbers to perform arithmetic operations on the list elements?
-
Jake over 13 yearsTested this and realised it does not work because the strings have whitespace and extra quote marks in them.
-
DSM over 13 yearsYou need to strip the extra space as well.
-
Mark Tolonen over 13 yearsTested with a cut-and-paste of the OP's example? It didn't work as is.
-
Mark Tolonen over 13 yearsDoes not work with OP's exact example. Zeroes aren't "unnecessary".
float
can't represent all decimals exactly in binary. But see Mark Byers answer. -
Jake over 13 years@Mark, I've no idea what I did differently yesterday but it's not working now. Perhaps the question has been edited since I copied it.
-
tehfink over 5 yearsDoesn't this method lose the last 2 decimal places, resulting in a larger number?
-
freerunner over 5 years
price_in_decimal = Decimal(price.replace(',','.'))
This is what @Nids intended I suppose. Replacing the , with . -
Victor about 4 years@freerunner exactly!