How to read in floats from a file?
49,177
Solution 1
Assuming there's one float per line:
with open("myfile") as f:
floats = map(float, f)
# change floats
with open("myfile", "w") as f:
f.write("\n".join(map(str, floats)))
If you want more control with formatting, use the format
method of string. For instance, this will only print 3 digits after each period:
f.write("\n".join(map("{0:.3f}".format, floats)))
Solution 2
The "float()" function accepts strings as input and converts them into floats.
>>> float("123.456")
123.456
Solution 3
def get_numbers():
with open("yourfile.txt") as input_file:
for line in input_file:
line = line.strip()
for number in line.split():
yield float(number)
Then just write them back when your done
and as a shorter version (not tested, written from head)
with open("yourfile.txt") as input_file:
numbers = (float(number) for number in (line for line in (line.split() for line in input_file)))
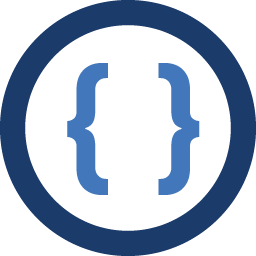
Author by
Admin
Updated on May 20, 2020Comments
-
Admin almost 4 years
How can I open a file and read in the floats from the file, when it is in string format, in Python? I would also like to change the values of the each float and rewrite the file over with the new values.