Writing variables to new line of txt file in python
26,831
Solution 1
You just need to specify the newline character as a string:
Eg:
with open("file.txt", "w") as att_file:
for item in list:
att_file.write(attribute + "\n")
Solution 2
try this:
att_file.write(attribute+"\n")
note :attribute must be some variable and must be string type
your code will look like this:
with open("file.txt", "w") as att_file:
for item in list:
att_file.write(item+"\n")
with
should be before for
, else every time you are opening file with write mode, it will omit the
previous write
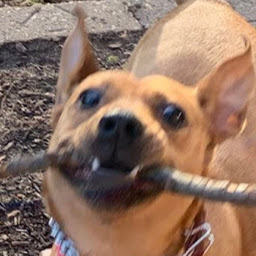
Author by
Shane
I enjoy learning new coding concepts, and bettering myself in my ability to find innovative code-based solutions to everyday problems.
Updated on June 13, 2020Comments
-
Shane almost 4 years
From other posts, I've learned that '\n' signifies a new line when adding to a txt file. I'm trying to do just this, but I can't figure out the right syntax when an attribute is right before the new line.
My code I'm trying is like this:
for item in list: with open("file.txt", "w") as att_file: att_file.write(variable\n)
As you can probably see, I'm trying to add the variable for each item in the list to a new line in a txt file. What's the correct way to do this?