How to split a string input and append to a list? Python
Solution 1
for i in words:
list_of_food = list_of_food.append(i)
You should change this just to
for i in words:
list_of_food.append(i)
For two different reasons. First, list.append()
is an in-place operator, so you don't need to worry about reassigning your list when you use it. Second, when you're trying to use a global variable inside a function, you either need to declare it as global
or never assign to it. Otherwise, the only thing you'll be doing is modifying a local. This is what you're probably trying to do with your function.
def split_food(input):
global list_of_food
#split the input
words = input.split()
for i in words:
list_of_food.append(i)
However, because you shouldn't use globals unless absolutely necessary (it's not a great practice), this is the best method:
def split_food(input, food_list):
#split the input
words = input.split()
for i in words:
food_list.append(i)
return food_list
Solution 2
>>> text = "What can I say about this place. The staff of these restaurants is nice and the eggplant is not bad.'
>>> txt1 = text.split('.')
>>> txt2 = [line.split() for line in txt1]
>>> new_list = []
>>> for i in range(0, len(txt2)):
l1 = txt2[i]
for w in l1:
new_list.append(w)
print(new_list)
Solution 3
Use the "extend" keyword. This aggregates two lists together.
list_of_food = []
def split_food(input):
#split the input
words = input.split()
list_of_food.extend(words)
print list_of_food
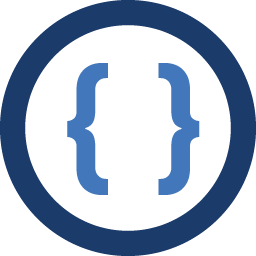
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I want to ask the user what foods they have ate, then split that input up into a list. Right now, the code is spitting out just empty brackets.
Also, this is my first post on here, so I apologize in advance for any formating errors.
list_of_food = [] def split_food(input): #split the input words = input.split() for i in words: list_of_food = list_of_food.append(i) print list_of_food
-
chepner over 9 yearsOr better, skip the for loop and use
list_of_food.extend(words)
. -
Robert Moskal over 9 yearsThere's no need for the list_of_food at all is there? words will be a list as soon as you do the split.
-
user3012759 over 9 years@Robert Moskal if you want to return at all that is, append will modify your list anyway
-
jps over 6 years
split()
already returns a list, so you iterate over one list to copy element by element to another list