Validating user input strings in Python
Solution 1
You are testing if the user input is yes
or no
. Add a not
:
while userInput not in ['yes', 'no']:
Ever so slightly faster and closer to your intent, use a set:
while userInput not in {'yes', 'no'}:
What you used is userInput in ['yes', 'no']
, which is True
if userInput
is either equal to 'yes'
or 'no'
.
Next, use a boolean to set endProgram
:
endProgram = userInput == 'no'
Because you already verified that userInput
is either yes
or no
, there is no need to test for yes
or no
again to set your flag variable.
Solution 2
def transaction():
print("Do the transaction here")
def getuserinput():
userInput = "";
print("Start")
while "no" not in userInput:
#Prompt for a new transaction
userInput = input("Would you like to start a new transaction?")
userInput = userInput.lower()
if "no" not in userInput and "yes" not in userInput:
print("yes or no please")
if "yes" in userInput:
transaction()
print("Good bye")
#Main program
getuserinput()
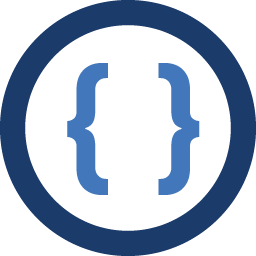
Admin
Updated on September 12, 2020Comments
-
Admin over 3 years
So I've searched just about every permutation of the words "string", "python", "validate", "user input", and so on, but I've yet to come across a solution that's working for me.
My goal is to prompt the user on whether or not they want to start another transaction using the strings "yes" and "no", and I figured that string comparison would be a fairly easy process in Python, but something just isn't working right. I am using Python 3.X, so input should be taking in a string without using raw input, as far as I understand.
The program will always kick back invalid input, even when entering 'yes' or 'no', but the really weird thing is that every time I enter a a string > 4 characters in length or an int value, it will check it as valid positive input and restart the program. I have not found a way to get valid negative input.
endProgram = 0; while endProgram != 1: #Prompt for a new transaction userInput = input("Would you like to start a new transaction?: "); userInput = userInput.lower(); #Validate input while userInput in ['yes', 'no']: print ("Invalid input. Please try again.") userInput = input("Would you like to start a new transaction?: ") userInput = userInput.lower() if userInput == 'yes': endProgram = 0 if userInput == 'no': endProgram = 1
I have also tried
while userInput != 'yes' or userInput != 'no':
I would greatly appreciate not only help with my problem, but if anyone has any additional information on how Python handles strings that would be great.
Sorry in advance if someone else has already asked a question like this, but I did my best to search.
Thanks all!
~Dave
-
Admin about 11 yearsWow. Such a simple mistake. Thanks for the prompt reply. I guess sometimes you just need a second pair of eyes to spot things.
-
Admin about 11 yearsAs a side note, could you help me learn why my original method of while userInput != 'yes' or userInput != 'no': wasn't working?
-
Martijn Pieters about 11 years@user2398870: If
userInput
is set to'yes'
, then!= 'no'
is True. That is not what you wanted to test. :-) Changing theor
toand
would make that version work.