Python: Creating a List of the First n Fibonacci Numbers
14,713
Solution 1
Try this, a recursive implementation that returns a list of numbers by first calculating the list of previous values:
def fib(n):
if n == 0:
return [0]
elif n == 1:
return [0, 1]
else:
lst = fib(n-1)
lst.append(lst[-1] + lst[-2])
return lst
It works as expected:
fib(8)
=> [0, 1, 1, 2, 3, 5, 8, 13, 21]
Solution 2
Here's one way using generators....
def fib(n):
a, b = 0, 1
for _ in xrange(n):
yield a
a, b = b, a + b
print list(fib(8)) #prints: [0, 1, 1, 2, 3, 5, 8, 13]
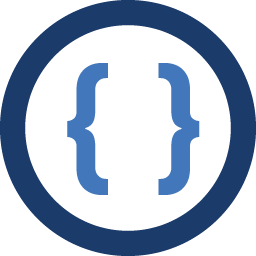
Author by
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
I am new to Python and to these forums.
My question is: How can I create a list of
n
Fibonacci numbers in Python?So far, I have a function that gives the
nth
Fibonacci number, but I want to have a list of the firstn
Fib. numbers for future work.For example:
fib(8) -> [0,1,1,2,3,5,8,13]