Python Fibonacci Generator
Solution 1
I would use this method:
Python 2
a = int(raw_input('Give amount: '))
def fib(n):
a, b = 0, 1
for _ in xrange(n):
yield a
a, b = b, a + b
print list(fib(a))
Python 3
a = int(input('Give amount: '))
def fib(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
print(list(fib(a)))
Solution 2
You are giving a
too many meanings:
a = int(raw_input('Give amount: '))
vs.
a = fib()
You won't run into the problem (as often) if you give your variables more descriptive names (3 different uses of the name a
in 10 lines of code!):
amount = int(raw_input('Give amount: '))
and change range(a)
to range(amount)
.
Solution 3
Since you are writing a generator, why not use two yields, to save doing the extra shuffle?
import itertools as it
num_iterations = int(raw_input('How many? '))
def fib():
a,b = 0,1
while True:
yield a
b = a+b
yield b
a = a+b
for x in it.islice(fib(), num_iterations):
print x
.....
Solution 4
Really simple with generator:
def fin(n):
a, b = 0, 1
for i in range(n):
yield a
a, b = b, a + b
ln = int(input('How long? '))
print(list(fin(ln))) # [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ...]
Solution 5
Python is a dynamically typed language. the type of a variable is determined at runtime and it can vary as the execution is in progress. Here at first, you have declared a to hold an integer type and later you have assigned a function to it and so its type now became a function.
you are trying to apply 'a' as an argument to range() function which expects an int arg but you have in effect provided a function variable as argument.
the corrected code should be
a = int(raw_input('Give amount: '))
def fib():
a, b = 0, 1
while 1:
yield a
a, b = b, a + b
b = fib()
b.next()
for i in range(a):
print b.next(),
this will work
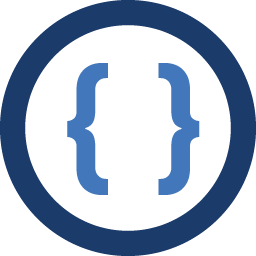
Admin
Updated on November 17, 2021Comments
-
Admin over 2 years
I need to make a program that asks for the amount of Fibonacci numbers printed and then prints them like 0, 1, 1, 2... but I can't get it to work. My code looks the following:
a = int(raw_input('Give amount: ')) def fib(): a, b = 0, 1 while 1: yield a a, b = b, a + b a = fib() a.next() 0 for i in range(a): print a.next(),
-
AgentRev over 4 years
fib(0)
doesn't yield anything, which can be fixed usingrange(n+1)
instead. -
saeed foroughi over 4 yearsonly code answers are discouraged here. It is encouraged to add some details to how this code will fix the problem.
-
Neosapien about 4 yearsI rewrote this by using a=0, b=1, a=b and b=a+b because I was uncomfortable with that notation but it gave completely different answers. Am I missing something?
-
Jyothish Bhaskaran about 4 yearsI am gettting error on this File "D:\Projects\Python\fibonacci.py", line 18, in <module> b.next() AttributeError: 'generator' object has no attribute 'next'
-
Jyothish Bhaskaran about 4 yearsI found it for Python 3, it is b.__next__(). Sorry :) and Thanks
-
Tomerikoo over 2 yearsYeah but the question is asking to print the first
n
numbers, not then
th number...