Python: how to "kill" a class instance/object?
Solution 1
In general:
- Each binding variable -> object increases internal object's reference counter
-
there are several usual ways to decrease reference (dereference object -> variable binding):
- exiting block of code where variable was declared (used for the first time)
- destructing object will release references of all attributes/method variable -> object references
- calling
del variable
will also delete reference in the current context
after all references to one object are removed (counter==0) it becomes good candidate for garbage collection, but it is not guaranteed that it will be processed (reference here):
CPython currently uses a reference-counting scheme with (optional) delayed detection of cyclically linked garbage, which collects most objects as soon as they become unreachable, but is not guaranteed to collect garbage containing circular references. See the documentation of the gc module for information on controlling the collection of cyclic garbage. Other implementations act differently and CPython may change. Do not depend on immediate finalization of objects when they become unreachable (ex: always close files).
how many references on the object exists, use sys.getrefcount
module for configure/check garbage collection is gc
GC will call object.__ del__ method when destroying object (additional reference here)
some immutable objects like strings are handled in a special way - e.g. if two vars contain same string, it is possible that they reference the same object, but some not - check identifying objects, why does the returned value from id(...) change?
id of object can be found out with builtin function id
module memory_profiler looks interesting - A module for monitoring memory usage of a python program
there is lot of useful resources for the topic, one example: Find all references to an object in python
Solution 2
You cannot force a Python object to be deleted; it will be deleted when nothing references it (or when it's in a cycle only referred to be the items in the cycle). You will have to tell your "Mastermind" to erase its reference.
del somemastermind.roaches[n]
Solution 3
for i,roach in enumerate(roachpopulation_list)
if roach.hunger == 100
del roachpopulation_list[i]
break
Remove the instance by deleting it from your population list (containing all the roach instances.
If your Roaches are Sprites created in Pygame, then a simple command of .kill would remove the instance.
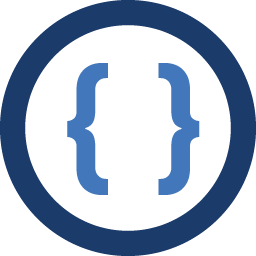
Admin
Updated on February 01, 2020Comments
-
Admin over 4 years
I want a Roach class to "die" when it reaches a certain amount of "hunger", but I don't know how to delete the instance. I may be making a mistake with my terminology, but what I mean to say is that I have a ton of "roaches" on the window and I want specific ones to disappear entirely.
I would show you the code, but it's quite long. I have the Roach class being appended into a Mastermind classes roach population list.
-
Jo Mo over 3 yearscan you also not do the numeration, and just
roachpopulation_list.remove(roach)
, thendel roach
? It seems to me both approaches should be equivalent, but I'm not sure -
Beatrix Kidco over 3 yearsYou could use any of the functions in Python to remove the instance from the list, like delete, remove, pop etc. Once an instance is no longer stored in a list or dictionary or variable etc, it's forgotten.