Python: How to use user input to close or continue a while loop
You are use Python 2.x. input()
tries to run the input as a valid Python expression. When you enter a string, it tries to look for it in the namespace, if it is not found it throws an error:
NameError: name 'yes' is not defined
You should not use input
to receive unfiltered user input, it can be dangerous. Instead, use raw_input()
that returns a string:
play = True
while play:
hours = float(raw_input("Please enter the number of hours worked this week: "))
rate = float(raw_input("Please enter your hourly rate of pay: "))
#if less than 40 hrs
if hours <= 40:
pay = hours * rate
print "Your pay for this week is",pay
#overtime 54hrs or less
elif hours > 40 < 54:
timeandhalf = rate * 1.5
pay = (40 * hours * rate) + ((hours - 40) * timeandhalf)
print "Your pay for this week is",pay
#doubletime more than 54hrs
elif hours > 54:
timeandhalf = rate * 1.5
doubletime = rate * 2
pay = (40 * hours * rate) + ((hours - 40) * timeandhalf) + ((hours - 54) * doubletime)
print "Your pay for this week is",pay
answer = raw_input("Would you like to try again?: ").lower()
while True:
if answer == 'yes':
play = True
break
elif answer == 'no':
play = False
break
else:
answer = raw_input('Incorrect option. Type "YES" to try again or "NO" to leave": ').lower()
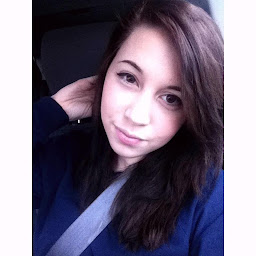
Savannah Nicole
Updated on June 05, 2022Comments
-
Savannah Nicole almost 2 years
I am new to python, and still trying to figure out the basics. I've looked for an answer online, but no solutions seem to work for me. I don't know if I'm missing something small, or my total structure is wrong. The calculation portion of my code works like it's supposed to.
My problem is, I can't figure out how to ask the user to input yes or no resulting in:
(the answer YES restarting the loop so the user can try another calculation)
(the answer NO closing the loop/ending the program)
Please let me know what you suggest!
play = True while play: hours = float(input("Please enter the number of hours worked this week: ")) rate = float(input("Please enter your hourly rate of pay: ")) #if less than 40 hrs if hours <= 40: pay = hours * rate print "Your pay for this week is",pay #overtime 54hrs or less elif hours > 40 < 54: timeandhalf = rate * 1.5 pay = (40 * hours * rate) + ((hours - 40) * timeandhalf) print "Your pay for this week is",pay #doubletime more than 54hrs elif hours > 54: timeandhalf = rate * 1.5 doubletime = rate * 2 pay = (40 * hours * rate) + ((hours - 40) * timeandhalf) + ((hours - 54) * doubletime) print "Your pay for this week is",pay answer = (input("Would you like to try again?: ")) if str(answer) == 'yes': play = True else: play = False