Python inheritance - calling base class methods inside child class?
Solution 1
Usually, you do this when you want to extend the functionality by modifiying, but not completely replacing a base class method. defaultdict
is a good example of this:
class DefaultDict(dict):
def __init__(self, default):
self.default = default
dict.__init__(self)
def __getitem__(self, key):
try:
return dict.__getitem__(self, key)
except KeyError:
result = self[key] = self.default()
return result
Note that the appropriate way to do this is to use super
instead of directly calling the base class. Like so:
class BlahBlah(someObject, someOtherObject):
def __init__(self, *args, **kwargs):
#do custom stuff
super(BlahBlah, self).__init__(*args, **kwargs) # now call the parent class(es)
Solution 2
It completely depends on the class and method.
If you just want to do something before/after the base method runs or call it with different arguments, you obviously call the base method in your subclass' method.
If you want to replace the whole method, you obviously do not call it.
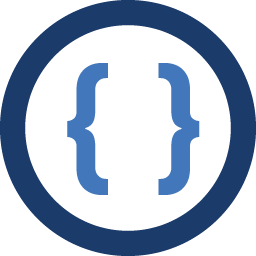
Admin
Updated on May 02, 2020Comments
-
Admin about 4 years
It baffles me how I can't find a clear explanation of this anywhere. Why and when do you need to call the method of the base class inside the same-name method of the child class?
class Child(Base): def __init__(self): Base.__init__(self) def somefunc(self): Base.somefunc(self)
I'm guessing you do this when you don't want to completely overwrite the method in the base class. is that really all there is to it?
-
Amber about 12 yearsAs a side note, however, you should always call the parent class'
__init__
. -
Admin about 12 yearsWhat's so great about this "super" functions?
-
Joel Cornett about 12 years@user1369281: Well, they're not always great. If you use them consistently however, they make inheritance trees much easier to work with. What if, for example, I wanted to change my
defaultdict
to a default ordered dict. If I usedsuper()
, the only thing I would have to change in my code would be the class definition. All thosesuper
's automatically refer to whatever base class my current class is inheriting from. It facilitates black box programming. -
casey over 10 yearsI like this because it reminds you (or teaches) that your class can inherit multiple classes. So if your parent class is from a lib you can't modify and doesn't inherit object already, you can just add
object
to your class.