Python passing list as argument
Solution 1
Everything is a reference in Python. If you wish to avoid that behavior you would have to create a new copy of the original with list()
. If the list contains more references, you'd need to use deepcopy()
def modify(l):
l.append('HI')
return l
def preserve(l):
t = list(l)
t.append('HI')
return t
example = list()
modify(example)
print(example)
example = list()
preserve(example)
print(example)
outputs
['HI']
[]
Solution 2
The easiest way to modify the code will be add the [:] to the function call.
def function(y):
y.append('yes')
return y
example = list()
function(example[:])
print(example)
Solution 3
"Why would it return ['yes']
"
Because you modified the list, example
.
"even though i am not directly changing the variable 'example'."
But you are, you provided the object named by the variable example
to the function. The function modified the object using the object's append
method.
As discussed elsewhere on SO, append
does not create anything new. It modifies an object in place.
See Why does list.append evaluate to false?, Python append() vs. + operator on lists, why do these give different results?, Python lists append return value.
and how could I modify the code so that 'example' is not effected by the function?
What do you mean by that? If you don't want example
to be updated by the function, don't pass it to the function.
If you want the function to create a new list, then write the function to create a new list.
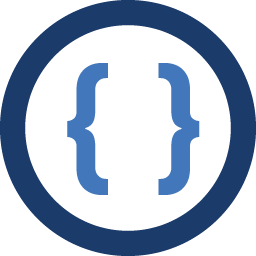
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
If i were to run this code:
def function(y): y.append('yes') return y example = list() function(example) print(example)
Why would it return ['yes'] even though i am not directly changing the variable 'example', and how could I modify the code so that 'example' is not effected by the function?