Python recursive function counting the user
Solution 1
def count_users(group):
count = 0
for member in get_members(group):
#count += 1
if is_group(member):
count += count_users(member)
else:
count+=1
return count
print(count_users("sales")) # Should be 3
print(count_users("engineering")) # Should be 8
print(count_users("everyone")) # Should be 18
Solution 2
The problem lies in your for loop. Currently you increase your count by 1 for each "member" of the group, even if that member is a group itself.
Instead move this line into a new else branch after the if is_group(member), so it will only be increased by either the number of members if member
is a group, or 1 if member
is not a group.
Solution 3
def count_users(group):
count = 0
for member in get_members(group):
count += 1
if is_group(member):
count += count_users(member)-1
return count
print(count_users("sales")) # Should be 3
print(count_users("engineering")) # Should be 8
print(count_users("everyone")) # Should be 18
Solution 4
Here's the correct solution :
def count_users(group):
count = 0
for member in get_members(group):
count += 1
if is_group(member):
count += count_users(member)-1
return count
print(count_users("sales"))
# Should be 3
print(count_users("engineering"))
# Should be 8
print(count_users("everyone"))
# Should be 18
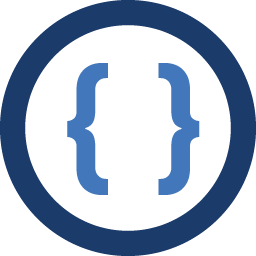
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
The count_users function recursively counts the amount of users that belong to a group in the company system, by going through each of the members of a group and if one of them is a group, recursively calling the function and counting the members. But it has a bug! Can you spot the problem and fix it?
def count_users(group): count = 0 for member in get_members(group): count += 1 if is_group(member): count += count_users(member) return count print(count_users("sales")) # Should be 3 print(count_users("engineering")) # Should be 8 print(count_users("everyone")) # Should be 18
-
Brian about 4 yearsWhile this code may solve the question, including an explanation of how and why this solves the problem would really help to improve the quality of your post, and probably result in more up-votes. Remember that you are answering the question for readers in the future, not just the person asking now. Please edit your answer to add explanations and give an indication of what limitations and assumptions apply.