Qt: how to make mainWindow automatically resize when centralwidget is resized?
You can reimplement QMainWindow::event()
to resize the window automatically whenever the size of the central widget changes:
bool MyMainWindow::event(QEvent *ev) {
if(ev->type() == QEvent::LayoutRequest) {
setFixedSize(sizeHint());
}
return result = QMainWindow::event(ev);
}
You also have to use setFixedSize()
for the central widget instead of just resize()
. The latter is almost useless when the widget is placed inside a layout (which is a QMainWindowLayout
here).
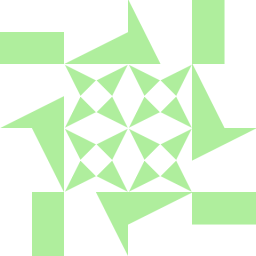
Mat
Updated on June 13, 2022Comments
-
Mat 7 months
I would like to have my CentralWidget a certain size. What do I need to do to make my mainWindow resize along it's central widget? here the code that doesn't work:
int main (int argc, char **argv) { QApplication app(argc, argv); QGLFormat glFormat; glFormat.setVersion(4,2); glFormat.setProfile( QGLFormat::CompatibilityProfile); QGLWidget* render_qglwidget = new MyWidget(glFormat); QGLContext* glContext = (QGLContext *) render_qglwidget->context(); glContext->makeCurrent(); QMainWindow* mainWindow = new MyMainWindow(); render_qglwidget->resize(720, 486); mainWindow->setSizePolicy(QSizePolicy(QSizePolicy::Expanding,QSizePolicy::Expanding)); mainWindow->setCentralWidget(render_qglwidget); render_qglwidget->resize(720, 486); mainWindow->show(); return app.exec(); }
the window that opens will be very small.
i can set the size of the mainwindow using
mainWindow->resize(720, 486);
and the centralwidget will also change it's size. but the central widget will be slightly squashed because the toolbar of the mainWindow also lies within those 486 pixels.
How to let the mainWindow resize automatically?
-
Mat almost 11 yearsthanks. if i add mainWindow->setFixedSize(hint.width(), hint.height()); for some reasons the window never opens
-
alexisdm almost 11 years@Mat Did you call set
mainWindow->setFixedSize(hint)
aftersetCentralWidget
? -
Mat almost 11 yearsthx. if i put setWindowFlags(Qt::FramelessWindowHint) i cannot resize by mouse, but i also cannot move the window arround. i would like to forbid resizing by mouse but allow moving the window arround. how to do that?
-
Mat almost 11 yearsyes - 1. mainWindow->setCentralWidget, 2. centralWidget->resize(), 3. mainwindow->setFixedSize(hint), 4.mainWindow->show(). the 3. step makes the window not appear (it appears if i comment out step 3)
-
Mat almost 11 yearsthis makes the mainwindow pop up for a short moment (small) and close instantly
-
alexisdm almost 11 years@Mat I edited my answer, using setFixedSize should also work with NFRCR solution.