Quaternion to Matrix using glm
22,506
Solution 1
Add the include:
#include <glm/gtx/quaternion.hpp>
And fix the namespace of toMat4
:
glm::mat4 RotationMatrix = glm::toMat4(myQuat);
glm::toMat4()
exists in the gtx/quaternion.hpp
file, which you can see only has the glm
namespace.
Also as a side note, as of C++14, nested namespaces (e.g. glm::quaternion::toMat4) are not allowed.
Solution 2
In addition to meepzh's answer, it can also be done like this:
glm::mat4 RotationMatrix = glm::mat4_cast(myQuat);
which does not require #include <glm/gtx/quaternion.hpp>
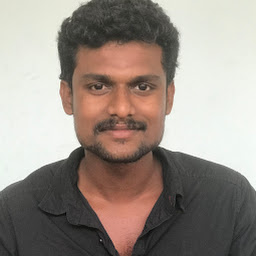
Author by
Vishnu Murali
Updated on July 09, 2022Comments
-
Vishnu Murali almost 2 years
I am trying to convert quat in glm to mat4.
My code is :
#include <iostream> #include<glm/glm.hpp> #include<glm/gtc/quaternion.hpp> #include<glm/common.hpp> using namespace std; int main() { glm::mat4 MyMatrix=glm::mat4(); glm::quat myQuat; myQuat=glm::quat(0.707107,0.707107,0.00,0.000); glm::mat4 RotationMatrix = quaternion::toMat4(myQuat); for(int i=0;i<4;++i) { for(int j=0;j<4;++j) { cout<<RotationMatrix[i][j]<<" "; } cout<<"\n"; } return 0; }
When i run the program it shows the error "error: ‘quaternion’ has not been declared".
Can anyone help me with this?
-
scry over 5 yearsNested namespace definitions are not allowed in C++14, but there are none defined in the question... anyway, they are allowed in C++17.
-
meepzh about 4 yearsIt was mentioned in the comments, but in hindsight I should have responded there.
-
user3882729 over 2 yearsOn Fedora-32 desktop (Linux) it does require the above include.