React-Bootstrap Dropdown with Input won't stay open
Solution 1
I managed to get it working by having a custom onToggle for dropdown that does nothing if click comes from input element. I basically ended up with something like this:
So something like this:
var React = require('react');
var ReactBootstrap = require('react-bootstrap'),
Dropdown = ReactBootstrap.Dropdown,
DropdownToggle = Dropdown.Toggle,
DropdownMenu = Dropdown.Menu,
Input = ReactBootstrap.Input,
MenuItem = ReactBootstrap.MenuItem;
module.exports = React.createClass({
displayName: 'DropdownWithInput',
setValue: function(e) {
var value = e.target.value;
this.setState({value: value});
},
onSelect: function(event, value) {
this.setState({value: value});
},
inputWasClicked: function() {
this._inputWasClicked = true;
},
onToggle: function(open) {
if (this._inputWasClicked) {
this._inputWasClicked = false;
return;
}
this.setState({open: open});
},
render: function() {
return (
<Dropdown id={this.props.id} open={this.state.open} onToggle={this.onToggle}
className="btn-group-xs btn-group-default">
<DropdownToggle bsStyle="default" bsSize="xs" className="dropdown-with-input dropdown-toggle">
Dropdown with input
</DropdownToggle>
<DropdownMenu>
<Input
type="text"
ref="inputElem"
autoComplete="off"
name={this.props.name}
placeholder="Type something here"
onSelect={this.inputWasClicked}
onChange={this.setValue}
/>
<MenuItem divider key={this.props.id + '-dropdown-input-divider'}/>
<MenuItem eventKey={1} onSelect={this.onSelect}>One</MenuItem>
<MenuItem eventKey={2} onSelect={this.onSelect}>Two</MenuItem>
<MenuItem eventKey={3} onSelect={this.onSelect}>Three</MenuItem>
</DropdownMenu>
</Dropdown>
);
}
});
Hope this works for you as well.
Solution 2
This capability is a relatively new feature for React-Bootstrap. Checkout the newer Dropdown Customization docs: http://react-bootstrap.github.io/components.html#btn-dropdowns-custom The last example of that section includes a dropdown with an input. The dropdown trigger looks like a link there, but you can also customize that.
Solution 3
I found a solution to this issue that worked pretty well for me. Inside the dropdown menu I had a a text input (not inside of a MenuItem).
<input onSelect={e => e.stopPropagation()} ... />
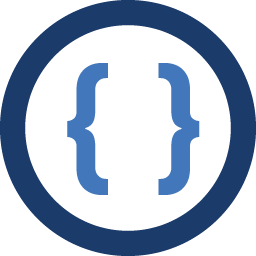
Admin
Updated on June 22, 2022Comments
-
Admin almost 2 years
I am using React-Bootstrap DropDown with an Input inside a MenuItem (otherwise the console yells at me Uncaught TypeError: Cannot read property 'focus' of undefined)
Okay so the drop down renders, and the input is inside a menu item (all is well) except when I click inside the input, the dropdown closes.
Here is my JSX
<Bootstrap.DropdownButton title={this.state.callIdTitle} id="callId"> <Bootstrap.MenuItem eventKey='1'> <input ref="callIdInput" type='text' eventKey='2' placeholder='Enter Call ID' /> </Bootstrap.MenuItem> </Bootstrap.DropdownButton>
Any pointing in the right direction is very much appreciated, I have been trying to solve this all day.
Thank you.
-
Sachin almost 6 yearsHere is my code still not work
<Dropdown id="myID"> <Dropdown.Toggle noCaret bsStyle="success" children={<i className="fas fa-ellipsis-h"></i>}/> <Dropdown.Menu> <input value="edit this text" type="text" name="notes" id="abc" onSelect={e => e.stopPropagation()} /> </Dropdown.Menu> </Dropdown>