React Router - Stay at the same page after refresh
Solution 1
Check that firebase does not interfares with the client side routes :P
You can use Index routes to achieve this.
You have your navigation i.e the layout of all pages in your app component so make it the root route.
Then you want your home route to be your default route so make it your Index route.
You need to import IndexRoute from react-router package (from which you import Route).
Add this-
import { Router, Route, IndexRoute } from 'react-router';
and then make your routes like below.
Use this-
<Provider store={store}>
<Router history={browserHistory}>
<Route path="/" component={App}>
<IndexRoute component={Home} />
<Route path="/home" component={Home}/>
<Route path="/allhotels" component={AllHotels}/>
<Route path="/addhotel" component={AddHotel} />
<Route path="/about" component={About} />
</Route>
<Route path="/signin" component={SignIn} />
<Route path="/signup" component={SignUp} />
</Router>
</Provider>, document.getElementById('root')
Solution 2
I found the reason of my problem. I use also Firebase in my project and it causes the problem. Thanks guys for help.
EDIT ======================================================================
Mabye I will write how I've fixed my problem and what was the reason of it.
So i was checking if user is logged in or not in auth method. And there if user was authorized I was pushing /
to browserHistory.
It was mistake because every refresh method was executing and then also redirection was called as well.
Solution is just to check if during executing auth method I'm on Signin page or not. If it is Signin page then I'm pushing /
to browserHistory but if not then just don't push anything.
firebaseApp.auth().onAuthStateChanged(user => {
if (user) {
let currentPathname = browserHistory.getCurrentLocation().pathname;
if( currentPathname === "/" || currentPathname === "/signin"){
browserHistory.push('/');
}
const { email } = user;
store.dispatch(logUser(email));
}
else {
browserHistory.replace('/signin');
}
})
I know that it's not pretty solution but it works and it was only home project which was created to learn react. (btw this project is using old react router 3.0 so probalby now using browserHistory is deprecated)
Solution 3
it's a Server-side vs Client-side issue check the following thread, it might give you some insights.. React-router urls don't work when refreshing or writting manually
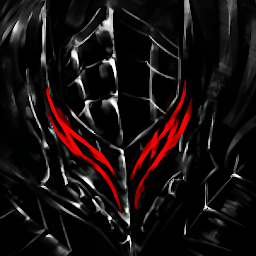
Skylin R
Updated on March 03, 2020Comments
-
Skylin R about 4 years
I'm learning React. I have page with 4 subpages and i use React Router to go through those pages. Everything works fine except for reloading page. When i go from page "home" to "about" or other it's ok, but when i refresh page then it render again page "about" for a second and then it change page to home (home is default/first page).I want it to stay at that page which is currently rendered, not go back to home after every refresh. There is my code in index.js file where i render whole app and use router:
<Provider store={store}> <Router path="/" history={browserHistory}> <Route path="/home" component={App}> <Route path="/" component={Home}/> <Route path="/allhotels" component={AllHotels}/> <Route path="/addhotel" component={AddHotel} /> <Route path="/about" component={About} /> </Route> <Route path="/signin" component={SignIn} /> <Route path="/signup" component={SignUp} /> </Router> </Provider>, document.getElementById('root')
In "App" i have Navigation and there i render rest of conent from Home, AllHotels etc.
There is code from App.jsx
class App extends Component { render() { return ( <div className="app-comp"> <Navigation /> <div> {this.props.children}</div> </div> ) } }
I also attach gif which shows my problem.
In backend i use Firebase if it's important.
Thanks in advance.
-
Skylin R almost 7 yearsIt's not workign now default render only App without any content and then Home button render Home content etc. But there is still the same problem.
-
Skylin R almost 7 yearsIt not helped :(
-
Simonov Dmitry almost 7 yearsHave you modified
output.publicPath
in your Webpack configuration? -
Skylin R almost 7 yearsI saw this solution in the internet but it not helps also. Acctualy it doesn't change anything. It works the same as my previous code.
-
Skylin R almost 7 yearsI have edited webpack js not configuration file, because i cant find it. Should i create it? I can't also find something like output public path. I haven't add webpack by myself, it was added automatically when I was creating project.
-
Simonov Dmitry almost 7 yearsThe
webpack.config.js
is at the root of the project. -
Arshmeet almost 7 yearshave you changed your routes with these? I am sure they will work. Please make sure you copy entire. I have removed path from your router component also which was causing this error.
-
Skylin R almost 7 yearsI've checked and in node modules i have folder webpack, but i don't have installed webpack because now i've install it. Unfortunately don't have webpack.config.js still. I've installed webpack from npmjs.com/package/webpack
-
Simonov Dmitry almost 7 yearsHow do you run project? What do you server use?
-
Skylin R almost 7 yearsYes, I've coppied entire snippet and paste it instead my code. But it not helps :(
-
Skylin R almost 7 yearsI use node, and i start project by npm start. I start creating app from here github.com/facebookincubator/create-react-app
-
Arshmeet almost 7 yearsWait let me see how can I share my code with you. Will it be any benefit if I share example from my own app?
-
Skylin R almost 7 yearsOf course it will help me when i see working solution, because for now i do what You said but really it not works. Maybe it's issue not from that file? Or just i have older version of React Router?
-
Arshmeet almost 7 yearsWhat version it is? v2 or v4
-
Skylin R almost 7 yearsI use version 3.0.1. Ok but when i do some changes or refresh page (but actually it's obvious) it also back to home from other pages like in my application.
-
Arshmeet almost 7 yearsLet us continue this discussion in chat.
-
Wingjam almost 6 years... and what was the problem/solution ?
-
Skylin R almost 6 years@Wingjam it was caused by my auth method where after checking user I was pushing '/' to browserHistory :P so with every app refresh I was checking if user is authorized and if he was then moved him to home directory ('/').
-
Rogelio almost 3 years@SkylinR you might have to eject your webpack config
npm run eject
oryarn run eject
ornpx react-scripts eject