React toggle like button
10,966
You could do something like the following.
Here is a codesandbox with a more complete version of the code.
import React from 'react';
class Likes extends React.Component {
constructor(props){
super(props);
this.state = {
likes: 124,
updated: false
};
}
updateLikes = () => {
if(!this.state.updated) {
this.setState((prevState, props) => {
return {
likes: prevState.likes + 1,
updated: true
};
});
} else {
this.setState((prevState, props) => {
return {
likes: prevState.likes - 1,
updated: false
};
});
}
}
render(){
return(
<div>
<p onClick={this.updateLikes}>Like</p>
<p>{this.state.likes}</p>
</div>
);
}
}
export default Likes;
Related videos on Youtube
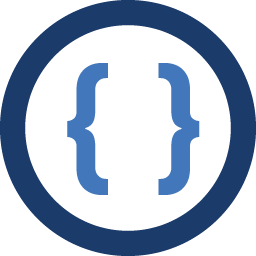
Author by
Admin
Updated on May 31, 2022Comments
-
Admin almost 2 years
I have a component with a state: {likes: 123} I display the number of likes next to a 'like' button. How do I implement a functionality to this button, so when I click it once, it adds to likes (so state.likes = 124), then if I want to click it a second time it goes back to previous state (state.likes = 123). Of course, it displays the correct number of likes at all times. Here's what I've got so far:
class ProfileInfo extends React.Component { constructor(props) { super(props); this.state = { likes: this.props.likes, }; } handleLike = () => { this.setState(prevState => ({ likes: prevState.likes + 1, })); } render() { return ( <div> <button className="like-button" onClick={this.handleLike}> Like </button> </div> ); } } export default ProfileInfo;
It just adds likes on and on.
-
Sami Ahmed Siddiqui over 6 yearsWhat have you tried so far? Please show your code.
-
Admin over 6 yearsI;ve edited my first post
-