ReactJS: How to dynamically render Material-UI's <MenuItem/> inside <DropDownMenu/>?
You've almost got it right. You have to map
over available colors and return a MenuItem
for each color:
const colors = ['white', 'blue', 'green'];
class ColorChanger extends Component {
constructor() {
super();
this.state = {
selectedColorValue: 1,
};
}
handleColorChange(event, index, value) {
console.log(`You have selected ${colors[value]} color`);
this.setState({
selectedColorValue: value
});
}
render() {
return (
<div>
<DropDownMenu value={this.state.selectedColorValue} onChange={this.handleColorChange}>
{colors.map((color, index) =>
<MenuItem key={index} value={index} primaryText={color} />
)}
</DropDownMenu>
</div>
);
}
}
map
(contrary to forEach
) returns an array where each element is the return value of predicate function. In your case it returns a <MenuItem />
.
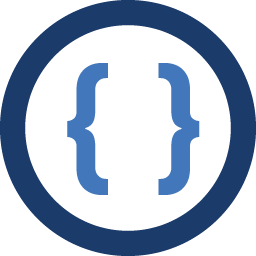
Admin
Updated on June 09, 2022Comments
-
Admin about 2 years
Using ReactJS + Material-UI, I have an array called
colors
and contains strings of different colors. Say for example the arraycolors
has 3 color strings: "white", "blue", "green. Then I would like to render each color string has a<MenuItem/>
inside a<DropDownMenu/>
(http://www.material-ui.com/#/components/dropdown-menu). And once a<MenuItem/>
is selected, I'd like to console log that particular color like, say chose "white": console.log("white").So I used .forEach yet the does not show any strings and it is empty. What could I be doing wrong?
Here is the code:
constructor() { super() this.state = { value: 1, } } dropDownColorChange(event, index, value) { this.setState({value: value}) //Not sure how to implement here dynamically based on array size. Would like to console.log the color string of the selected } render() { var colors = ["white", "blue", "green"] //would be able to handle any array size return ( <div> <DropDownMenu value={this.state.valueTwo} onChange={this.dropDownColorChange} > { <MenuItem value={1} primaryText="Select" /> colors.forEach(color => { <MenuItem primaryText={color}/> }) } </DropDownMenu> </div> ) }
Thank you
-
Kafo over 7 yearsYou will need to add
key
property to MenuItem so that React can track each MenuItem -
Konstantin Grushetsky over 7 yearsThat's correct, missed this one. The answer is updated.
-
Vivek Singh about 2 yearsI am doing the same but onchange event is not working on dynamically created menuitem any reason why.