Read image and determine if its corrupt C#
12,549
Try to create a GDI+ Bitmap from the file. If creating the Bitmap object fails, then you could assume the image is corrupt. GDI+ supports a number of file formats: BMP, GIF, JPEG, Exif, PNG, TIFF.
Something like this function should work:
public bool IsValidGDIPlusImage(string filename)
{
try
{
using (var bmp = new Bitmap(filename))
{
}
return true;
}
catch(Exception ex)
{
return false;
}
}
You may be able to limit the Exception
to just ArgumentException
, but I would experiment with that first before making the switch.
EDIT
If you have a byte[]
, then this should work:
public bool IsValidGDIPlusImage(byte[] imageData)
{
try
{
using (var ms = new MemoryStream(imageData))
{
using (var bmp = new Bitmap(ms))
{
}
}
return true;
}
catch (Exception ex)
{
return false;
}
}
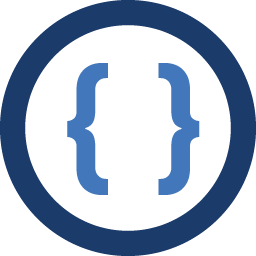
Author by
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
How do I determine if an image that I have as raw bytes is corrupted or not. Is there any opensource library that handles this issue for multiple formats in C#?
Thanks
-
Admin over 12 yearsOK. fine looks that is just what I needed! Thanks.
-
Harini Sampath about 9 yearsThis should be used with care. It's important that you release the Bitmap created with using. Else it is quite possible that you'd get OutOfMemory Exception while checking large number of files because the system ran out of resources and not because image is corrupt.
-
rsbarro about 9 years@Kip9000 When the code within the using statement is complete, the bitmap will be disposed. No need to explicitly dispose of it.
-
rsbarro about 9 yearsSee here for more info on using statements: msdn.microsoft.com/en-us/library/yh598w02.aspx
-
Harini Sampath about 9 yearsI was emphasizing that using 'using' is important. Your code is correct