regex over multiple lines in Groovy
Solution 1
(?m) makes the regex multiline - allows you to match beginning (^) and end ($) of string operators (in this case, to match the beginnings and ends of individual lines, rather than the whole string):
/(?m)(EXECUTE).*?;/
(?s) - dotall flag - makes the regex match newlines with . (dot) operators:
/(?s)(EXECUTE).*?;/
Solution 2
The following should work in Groovy.
def s = """
END IF;
EXECUTE IMMEDIATE ' CREATE INDEX #idx1
ON somename ( row_id,
something)';
IF v_sys_error <> 0 THEN
GOTO SQL_ERROR;
END IF;
"""
def expect = """
EXECUTE IMMEDIATE ' CREATE INDEX #idx1
ON somename ( row_id,
something)';
""".trim()
def exe = s =~ /(?ms)(EXECUTE.*?;)/
assert expect == exe[0][1]
Solution 3
I had the same question and the other two answeres pointed me in the right direction. In this particular case, you want to be able to use patterns (and select groups) that span multiple lines, i.e. you want the dot to also match newline characters. Default behaviour does not match newlines. That is why you need to use the dotall (s) flag:
/(?s)(EXECUTE).*?;/
This is how you specify flags in groovy patterns.
For testing different patterns and flags I found RegExr quite useful.
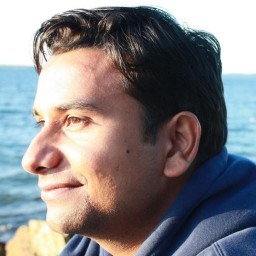
Comments
-
Omnipresent 11 months
I have a multiple line string like following:
END IF; EXECUTE IMMEDIATE ' CREATE INDEX #idx1 ON somename ( row_id, something)'; IF v_sys_error 0 THEN GOTO SQL_ERROR; END IF;
I wish to capture the part in bold (meaning everything from EXECUTE IMMEDIATE to next semicolon.
I have the following regex but how can I change it to work with multiple lines?
(EXECUTE).*;
-
Gaurav Khurana over 4 yearsThanks. Using m & s together give the benefit of making the pattern span across muiltiple lines
-
danski88 over 1 yearI thought that "regex match newlines with . (dot) operators" means that dot match only new line and not any other characters. Now I understand that it ADD new line to matching characters