Regex to escape special characters in Groovy
Note that [\W|_]
= [\W_]
, since |
is a non-word char. Also, it is advised to define regex with slashy strings since the backslashes inside them denote literal backslashes, the ones that are used to form regex escapes.
It seems you do not want to match spaces, so you need to subtract \s
from [\W_]
, use /[\W_&&[^\s]]/
regex.
Second, in the replacement part, you may use a single-quoted string literal to avoid interpolating $0
:
.replaceAll(specialCharRegex, '\\\\$0')
Else, escape the $
in the double quoted string literal:
.replaceAll(specialCharRegex, "\\\\\$0")
A slashy string also works as expected:
.replaceAll(specialCharRegex, /\\$0/)
See the online Groovy demo:
String specialCharRegex = /[\W_&&[^\s]]/;
println('test 1& test'.replaceAll(specialCharRegex, '\\\\$0')); // test 1\& test
println('test 1& test 2$'.replaceAll(specialCharRegex, "\\\\\$0")); // test 1\& test 2\$
println('test 1& test 2$ test 3%'.replaceAll(specialCharRegex, /\\$0/)); // test 1\& test 2\$ test 3\%
println('!"@#$%&/()=?'.replaceAll(specialCharRegex, /\\$0/)); // \!\"\@\#\$\%\&\/\(\)\=\?
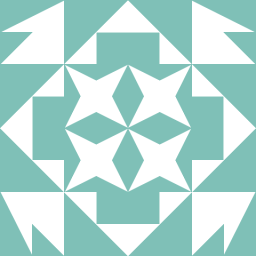
Dr3ko
Updated on June 05, 2022Comments
-
Dr3ko 12 months
I'm trying to use a regex to scape special characters, right now used it on Java and works perfect, it does exactly what I want
Scape any special character
however I tried this in Groovy but the same line doesn't work, as far I investaged it's because$
is reserved in Groovy, so far I tried this;Java: (Does the job)
String specialCharRegex = "[\\W|_]"; ... term = term.replaceAll(specialCharRegex, "\\\\$0"); ...
Groovy:
error
String specialCharRegex = "[\\W|_]"; ... term = term.replaceAll(specialCharRegex, "\\\\$0"); ...
error
String specialCharRegex = "[\\W|_]"; ... term = term.replaceAll(specialCharRegex, "\\\\\$0"); ...
error
String specialCharRegex = "[\\W|_]"; ... term = term.replaceAll(specialCharRegex, '\\\\$0'); ...
error
String specialCharRegex = "[\\W|_]"; ... term = term.replaceAll(specialCharRegex, '\\\\$1'); ...
I use
https://groovyconsole.appspot.com/
to test it.Output in Groovy should be:
Input: test 1& test Output: test 1\& test Input: test 1& test 2$ Output: test 1\& test 2\$ Input: test 1& test 2$ test 3% Output: test 1\& test 2\$ test 3\% Input: !"@#$%&/()=? Output: \!\"\@\#\$\%\&\/\(\)\=\?
-
Dr3ko almost 5 yearsOn ideon.com worked perfect even the ones with errors I posted, it may be the other page I was trying. Could you take a look and help if possible from this one; stackoverflow.com/questions/51159593/how-to-run-groovy-in-java trying to run in a Java (main) a method from a Groovy class.
-
MarkHu almost 5 yearsIt seems strange to use anything other than "quotes" to delimit a string, but I guess that is part of Groovy magic DSL. (Haven't seen
/regex/
slashes since my Perl olden-days.) -
Wiktor Stribiżew almost 5 years@MarkHu You may learn more about slashy strings here. There are even fancier dollar slashy strings in which you do not have to escape a
/
and$
. :)