reinitialize other javascript functions after loading a page with ajax pagination
When you load ajax-generated markup it will not retain the functionality it had before. In your example above, you're initialising things when the DOM is ready to be acted upon. In order to make sure any plugins, etc, are running after you perform the ajax request you need to reinitialise them.
Given your code sample above, I'd recommend a little restructuring. For example, you could create a function called init
which you could call to initialise certain plugins:
function init () {
$("#plugin-element").pluginName();
}
jQuery(document).ready(function () {
// Initialise the plugin when the DOM is ready to be acted upon
init();
});
And then following this, on the success callback of you ajax request, you can call it again which will reinitialise the plugins:
// inside jQuery(document).ready(...)
$.ajax({
type: 'GET',
url: 'page-to-request.html',
success: function (data, textStatus, jqXHR) {
// Do something with your requested markup (data)
$('#ajax-target').html(data);
// Reinitialise plugins:
init();
},
error: function (jqXHR, textStatus, errorThrown) {
// Callback for when the request fails
}
});
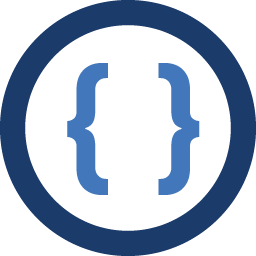
Admin
Updated on March 01, 2020Comments
-
Admin about 4 years
Apologies, a total newb here. How can I load other plugins, and let other separate scripts function after loading an ajax generated page? This is my curent code:
jQuery(document).ready(function($) { var $mainContent = $("load-content"), siteUrl = "http://" + top.location.host.toString(), url = ''; $(document).delegate("a[href^='"+siteUrl+"']:not([href*='/wp-admin/']):not([href*='/wp-login.php']):not([href$='/feed/'])", "click", function() { if($.browser.msie){ var myie="/"+this.pathname; location.hash = myie; //alert(location.hash); }else{ location.hash = this.pathname; } return false; }); $("#searchform").submit(function(e) { $search = $("#s").val(); $search = $.trim($search); $search = $search.replace(/\s+/g,'+'); location.hash = '?s='+$search; e.preventDefault(); }); $(window).bind('hashchange', function(){ url = window.location.hash.substring(1); if (!url) { return; } url = url + " #content"; $('html, body, document').animate({scrollTop:0}, 'fast'); $mainContent.fadeOut(500, function(){$('#content').fadeOut(500, function(){ $("#loader").show();});}).load(url, function() { $mainContent.fadeIn(500, function(){ $("#loader").hide(function(){ $('#content').fadeIn(500);});});}); }); $(window).trigger('hashchange'); });
How can embedded objects on pages retain their functionality? Mainly videos, slideshows and other media that use javascript like
vimeo
and
-
TimNguyenBSM almost 9 yearsbest answer on this topic I've found. Thanks. Does reinitializing cause noticeable performance issues?
-
6olden almost 9 yearsI'm really curious about the performance issues as well. I am working on a player which checks for a playlist on each new page load. It updates the array with each page. Somehow I can't get my plugin.pagePlaylist() to work. It works when I use the init(); function which contains that same pagePlaylist()... I'd rather only call the pagePlaylist().
-
Ĭsααc tիε βöss over 7 years@TimNguyenBSM, Nope there'll be no performance issues. Besides, if so, having an ajax loaded content without plugin initialization is childish and absurd.