Removing element from drop-down react-select
Adding and removing elements BOTH trigger the onChange
event with an array of currently selected values as a parameter.
For instance if my onChange
handler is, say, chooseAnimal
and my options are ["Mouse", "Cat", "Dog", "Duck"]
, when I select "Cat" then I will trigger chooseAnimal(["Cat"])
. If I then select "Mouse", I'll trigger chooseAnimal(["Cat", "Mouse"])
. And if I decide to remove "Cat", I'll trigger chooseAnimal(["Mouse"])
.
So say I have a simple state with a chosenAnimals
array, my chooseAnimal
handler would be very simple:
chooseAnimals = (chosenAnimals) => {
this.setState({ chosenAnimals });
}
In your own code, would the following work?
addMarket(markets) {
this.setState({ createNewDeliveryData: Object.assign({}, createNewDeliveryData, { markets }) });
// alternative form:
// let { createNewDeliveryData } = this.state;
// createNewDeliveryData.markets = markets;
// this.setState({ createNewDeliveryData });
}
Considering the structure of your market object, this is how you would set the options
in your Select tag:
options={this.props.metadata ? this.props.metadata["latest"].markets.map(m => return {value: m.id, label: m.default_name}) : []}
What map
does it that it'll iterate over your markets array and create a new array formed of value & label pairs for each element in the original markets array, and set the value to the id of that market element, and the label to the name of that same market element. Now the onChange handler should receive an array containing only market ids.
Related videos on Youtube
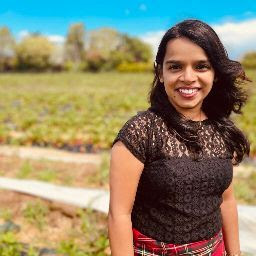
Nilakshi Naphade
Updated on May 29, 2022Comments
-
Nilakshi Naphade almost 2 years
I am using react-select with multi select option enabled. I want to add element after selecting option from the given list. Also if clicked on "x" (cross, which comes with selected option in input list) I want to delete that element from the selected input list. I am able to add element in the list using onChnage event, however, not sure how can I remove it. I thought react-select component should have some callback method to remove the element from the selected-list but I am not able to find it on github as well. The link that they have provided for multiselect is as below and I want something exactly like this: https://jedwatson.github.io/react-select/ (Check multi-select in this). I am attaching my html code snippet here:
createNewDeliveryData: { user_id: localStorage.getItem('userId'), //auth_token: localStorage.getItem('token'), is_listed: false, is_published: false, id:'', tags: [], languages: [], markets:[], order: 1, }, market = { default_name: "Deutschland" icon: "de" id:"44fc0d0c-d0ed-4013-924a-e15f4d589c83" } <If condition={!this.props.getDeliveryReqState}> <Select multi={true} name="form-field-name" options={this.props.metadata ? this.props.metadata["latest"].markets : []} labelKey="default_name" valueKey="id" onChange={this.addMarket} clearable={false} value={this.state.createNewDeliveryData.markets} /> </If> addMarket = (value) => { console.log("markets type",typeof(value)) this.setState({ createNewDeliveryData : { ...this.state.createNewDeliveryData, markets: value }, },function () { this.setValue({id:"markets", value:this.state.createNewDeliveryData.markets, error:null}) }); }
Can someone help me in this. It will be better if I can use react in-buil callback method instead of manually handling remove logic.
Thanks in advance.
-
Nilakshi Naphade over 6 yearsCan you explain it in my code? I am not sure how to implement it there. Just doing setState({value}) is not working for me. Showing nothing in input box. I have edited my code to show what object I have written in setState.
-
Nilakshi Naphade over 6 yearsI have one more issue regarding the same. I am updating working code above. But issue is, the "value" that I am setting using setValue function, have type "object", but I need string because otherwise my backend call is failing. I tried toString() function, but its not working.
-
Thomas Hennes over 6 years@NilakshiNaphade can you show me the structure of a market object?
-
Nilakshi Naphade over 6 yearsYes the problem is whole market object is being passed. I just want to pass id from it. I have updated above code to show how market object looks like. How can I pass only id as a value and not the whole object? I thought valueKey will work, but doesn't seem like working.
-
Thomas Hennes over 6 years@NilakshiNaphade couldn't find the additional code describing the structure of your market objects, but I've updated my answer regardless. Just replace
m.name
by whatever existing key in your market object represents the name of that market. -
Nilakshi Naphade over 6 yearsI didn't get your point. I want to display "default_name" in drop down and want to pass "id" as value.
-
Thomas Hennes over 6 years@NilakshiNaphade this is exactly what my code does. If you pass an array of
{ value: id, label: name }
objects, then names will be displayed in the drop down and ids will be passed by the onChange handler. -
Nilakshi Naphade over 6 yearsLet us continue this discussion in chat.