Removing elements from binding list
Solution 1
It's not working because you are working on a copy of the list which you created by calling ToList()
.
BindingList<T>
doesn't support RemoveAll()
: it's a List<T>
feature only, so:
IReadOnlyList<User> usersToRemove = UserList.Where(x => (x.id == ID)).
ToList();
foreach (User user in usersToRemove)
{
UserList.Remove(user);
}
We're calling ToList()
here because otherwise we'll enumerate a collection while modifying it.
Solution 2
You could try:
UserList = UserList.Where(x => x.id == ID).ToList();
If you use RemoveAll()
inside a generic class that you intend to be used to hold a collection of any type object, like this:
public class SomeClass<T>
{
internal List<T> InternalList;
public SomeClass() { InternalList = new List<T>(); }
public void RemoveAll(T theValue)
{
// this will work
InternalList.RemoveAll(x =< x.Equals(theValue));
// the usual form of Lambda Predicate
//for RemoveAll will not compile
// error: Cannot apply operator '==' to operands of Type 'T' and 'T'
// InternalList.RemoveAll(x =&gt; x == theValue);
}
}
This content is taken from here.
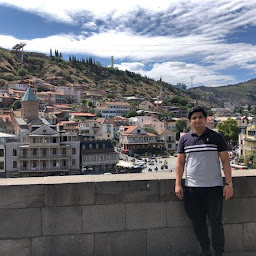
Rafay Zia Mir
Primarily a computational Physicist, secondarily a programmer.Love to work in both.Striving to learn learn and learn.I may be of little help but you can reach me at email: rafay_07[at]yahoo[dot]com
Updated on June 14, 2022Comments
-
Rafay Zia Mir almost 2 years
In one of my projects I'm trying to remove an item from a list where the id is equal to the given id.
I have a
BindingList<T>
calledUserList
.Lists have all the method
RemoveAll()
.Since I have a
BindingList<T>
, I use it like that:UserList.ToList().RemoveAll(x => x.id == ID )
However, my list contains the same number of items as before.
Why it's not working?