Replace '\n' in a string in Python 2.7
Solution 1
It is possible that your newlines are represented as \r\n
. In order to replace them you should do:
text.replace('\r\n', ' $ ')
For a portable solution that works on both UNIX-like systems (which uses \n
) and Windows (which uses \r\n
), you can substitute the text using a regex:
>>> import re
>>> re.sub('\r?\n', ' $ ', 'a\r\nb\r\nc')
'a $ b $ c'
>>> re.sub('\r?\n', ' $ ', 'a\nb\nc')
'a $ b $ c'
Solution 2
text = text.replace('\\n', '')
Solution 3
You can use splitlines.
lines = """Egg and Bacon;
Egg, sausage and Bacon
Egg and Spam;
Spam Egg Sausage and Spam;
Egg, Bacon and Spam;"""
print(" $ ".join(lines.splitlines()))
Egg and Bacon; $ Egg, sausage and Bacon $ Egg and Spam; $ Spam Egg Sausage and Spam; $ Egg, Bacon and Spam;
Or simply use rstrip and join on the file object without reading all into memory:
with open("in.txt") as f:
print(" $ ".join(line.rstrip() for line in f))
Egg and Bacon; $ Egg, sausage and Bacon $ Egg and Spam; $ Spam Egg Sausage and Spam; $ Egg, Bacon and Spam;
Which is a much more efficient solution than reading all the file into memory and using a regex. You should also always use with
to open your files as it closes them automatically.
rstrip will remove \n
\r\n
etc..
In [41]: s = "foo\r\n"
In [42]: s.rstrip()
Out[42]: 'foo'
In [43]: s = "foo\n"
In [44]: s.rstrip()
Out[44]: 'foo'
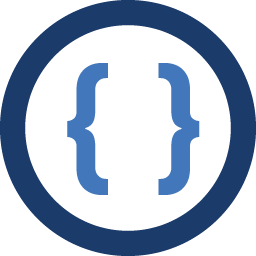
Admin
Updated on October 10, 2020Comments
-
Admin over 3 years
This is my file.txt:
Egg and Bacon; Egg, sausage and Bacon Egg and Spam; Spam Egg Sausage and Spam; Egg, Bacon and Spam;
I wanna convert the newLine '\n' to ' $ '. I just used:
f = open(fileName) text = f.read() text = text.replace('\n',' $ ') print(text)
This is my output:
$ Spam Egg Sausage and Spam;
and my output must be like:
Egg and Bacon; $ Egg, sausage and Bacon $ Egg ...
What am I doing wrong? I'm using
#-*- encoding: utf-8 -*-
Thank you.
-
juhist about 9 yearsAn additional comment: I would recommend doing first
text = text.replace('\r\n','\n')
and then doingtext = text.replace('\n', ' $ ')
. This works for all files, for the ones having'\n'
line separators and for the ones having'\r\n'
line separators. -
juhist about 9 yearsYes, I agree that your regex solution is better. Got already my upvote, though.