Replace a string in list of lists
Solution 1
Well, think about what your original code is doing:
example = [x.replace('\r\n','') for x in example]
You're using the .replace()
method on each element of the list as though it were a string. But each element of this list is another list! You don't want to call .replace()
on the child list, you want to call it on each of its contents.
For a nested list, use nested list comprehensions!
example = [["string 1", "a\r\ntest string:"],["string 1", "test 2: another\r\ntest string"]]
example = [[x.replace('\r\n','') for x in l] for l in example]
print example
[['string 1', 'atest string:'], ['string 1', 'test 2: anothertest string']]
Solution 2
example = [[x.replace('\r\n','') for x in i] for i in example]
Solution 3
In case your lists get more complicated than the one you gave as an example, for instance if they had three layers of nesting, the following would go through the list and all its sub-lists replacing the \r\n with space in any string it comes across.
def replace_chars(s):
return s.replace('\r\n', ' ')
def recursively_apply(l, f):
for n, i in enumerate(l):
if type(i) is list:
l[n] = recursively_apply(l[n], f)
elif type(i) is str:
l[n] = f(i)
return l
example = [[["dsfasdf", "another\r\ntest extra embedded"],
"ans a \r\n string here"],
['another \r\nlist'], "and \r\n another string"]
print recursively_apply(example, replace_chars)
Solution 4
The following example, iterate between lists of lists (sublists), in order to replace a string, a word.
myoldlist=[['aa bbbbb'],['dd myword'],['aa myword']]
mynewlist=[]
for i in xrange(0,3,1):
mynewlist.append([x.replace('myword', 'new_word') for x in myoldlist[i]])
print mynewlist
# ['aa bbbbb'],['dd new_word'],['aa new_word']
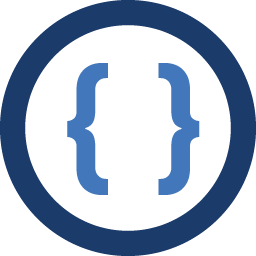
Admin
Updated on June 17, 2022Comments
-
Admin almost 2 years
I have a list of lists of strings like:
example = [["string 1", "a\r\ntest string:"],["string 1", "test 2: another\r\ntest string"]]
I'd like to replace the
"\r\n"
with a space (and strip off the":"
at the end for all the strings).For a normal list I would use list comprehension to strip or replace an item like
example = [x.replace('\r\n','') for x in example]
or even a lambda function
map(lambda x: str.replace(x, '\r\n', ''),example)
but I can't get it to work for a nested list. Any suggestions?