Retrieve location from Firebase and put marker on google map api for android
15,584
First : you nedd to change the structure to be like this :
Data
Location
-Kidp45TdInOM3Bsyu2b
latitude:19.772613777905196
longitude:-9.92741011083126
-KidsmTExZY2KjnS7S-b
latitude: 18.221073689785065
longitude: -6.573890447616577
-KidvmAgV0bm2uT_Pcdr
latitude: 14.44608051870992
longitude: -6.510856859385967
The way to do that is :
mSaveButton.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View view){
//change to refDatabase or mDatabase.child("Location")
DatabaseReference newPost = refDatabase.push();
//the push() command is already creating unique key
newPost.setValue(latlng);
}
});
And the code for put marker to the map from your refDatabase is :
@Override
public void onMapReady(GoogleMap googleMap) {
mMap = googleMap;
...
refDatabase.addChildEventListener(new ChildEventListener() {
@Override
public void onChildAdded(DataSnapshot dataSnapshot, String prevChildKey) {
LatLng newLocation = new LatLng(
dataSnapshot.child("latitude").getValue(Long.class),
dataSnapshot.child("longitude").getValue(Long.class)
);
mMap.addMarker(new MarkerOptions()
.position(newLocation)
.title(dataSnapshot.getKey()));
}
@Override
public void onChildChanged(DataSnapshot dataSnapshot, String prevChildKey) {}
@Override
public void onChildRemoved(DataSnapshot dataSnapshot) {}
@Override
public void onChildMoved(DataSnapshot dataSnapshot, String prevChildKey) {}
@Override
public void onCancelled(DatabaseError databaseError) {}
});
}
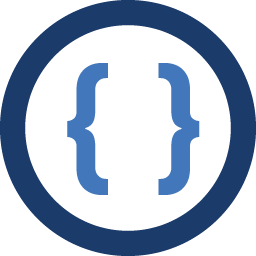
Author by
Admin
Updated on June 11, 2022Comments
-
Admin almost 2 years
I am trying to create app to store location on firebase when save button is pressed and retrieve locations from firebase and display all pins in the map. I have been able to save locations to firebase under Location child with latitude and longitude, but I have no idea how to get the values and pins. I tried to do it the way firebase has it on its manual but it didn't work. Can anyone help with the problem?
This is my code so far:
public class MapsActivity extends FragmentActivity implements OnMapReadyCallback { private GoogleMap mMap; private final static int MY_PERMISSION_FINE_LOCATION = 101; private Button mSaveButton; private DatabaseReference mDatabase; private DatabaseReference refDatabase; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_maps); // Obtain the SupportMapFragment and get notified when the map is ready to be used. SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager() .findFragmentById(R.id.map); mapFragment.getMapAsync(this); mSaveButton = (Button) findViewById(R.id.Savebtn); mDatabase = FirebaseDatabase.getInstance().getReference().child("Navigation"); refDatabase = FirebaseDatabase.getInstance().getReference().child("Location"); } @Override public void onMapReady(GoogleMap googleMap) { mMap = googleMap; mMap.setOnMapLongClickListener(new GoogleMap.OnMapLongClickListener() { @Override public void onMapLongClick(LatLng point) { // TODO Auto-generated method stub // added marker saved as marker and coordinates passed to latlng Marker marker = mMap.addMarker(new MarkerOptions().position(point)); final LatLng latlng = marker.getPosition(); mSaveButton.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View view){ DatabaseReference newPost = mDatabase.push(); newPost.child("Location").setValue(latlng); } }); } }); if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) { mMap.setMyLocationEnabled(true); } else { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { requestPermissions(new String[] {Manifest.permission.ACCESS_FINE_LOCATION}, MY_PERMISSION_FINE_LOCATION); } } } @Override public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) { super.onRequestPermissionsResult(requestCode, permissions, grantResults); switch (requestCode) { case MY_PERMISSION_FINE_LOCATION: if (grantResults[0] == PackageManager.PERMISSION_GRANTED) { if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) == PackageManager.PERMISSION_GRANTED) { mMap.setMyLocationEnabled(true); } }else { Toast.makeText(getApplicationContext(), "This requires location permissions to be granted", Toast .LENGTH_LONG).show(); finish(); } break; } } }
this is how the location been saved in firebase
Data -Kidp45TdInOM3Bsyu2b Location latitude:19.772613777905196 longitude:-9.92741011083126 -KidsmTExZY2KjnS7S-b Location latitude: 18.221073689785065 longitude: -6.573890447616577 -KidvmAgV0bm2uT_Pcdr Location latitude: 14.44608051870992 longitude: -6.510856859385967