Retrofit 2.2.0 Android API 24 javax.net.ssl.SSLHandshakeException: Handshake failed
11,836
Solution 1
The solution for me was adding more ciphers as acceptable for OkHttpClient. Since API 21, some TLS certificates are deprecated for Android. This might help:
ConnectionSpec spec = new
ConnectionSpec.Builder(ConnectionSpec.MODERN_TLS)
.tlsVersions(TlsVersion.TLS_1_2)
.cipherSuites(
CipherSuite.TLS_ECDHE_ECDSA_WITH_AES_128_GCM_SHA256,
CipherSuite.TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256,
CipherSuite.TLS_DHE_RSA_WITH_AES_128_GCM_SHA256)
.build();
OkHttpClient client = new OkHttpClient.Builder()
.connectionSpecs(Collections.singletonList(spec))
.build();
For more info please visit: https://github.com/square/okhttp/wiki/HTTPS
Solution 2
I have used it with 2.2.0
Create a trust manager that does not validate certificate chains
final TrustManager[] trustAllCerts = new TrustManager[]{
new X509TrustManager() {
@Override
public void checkClientTrusted(java.security.cert.X509Certificate[] chain, String authType) throws CertificateException {
}
@Override
public void checkServerTrusted(java.security.cert.X509Certificate[] chain, String authType) throws CertificateException {
}
@Override
public java.security.cert.X509Certificate[] getAcceptedIssuers() {
return new java.security.cert.X509Certificate[]{};
}
}
};
// Install the all-trusting trust manager
HttpLoggingInterceptor httpLoggingInterceptor = new HttpLoggingInterceptor();
httpLoggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder client = new OkHttpClient.Builder();
client.interceptors().add(httpLoggingInterceptor);
client.readTimeout(180, TimeUnit.SECONDS);
client.connectTimeout(180, TimeUnit.SECONDS);
KeyStore keyStore = KeyStore.getInstance(KeyStore.getDefaultType());
keyStore.load(null, null);
SSLContext sslContext = SSLContext.getInstance("TLS");
TrustManagerFactory trustManagerFactory = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm());
trustManagerFactory.init(keyStore);
KeyManagerFactory keyManagerFactory = KeyManagerFactory.getInstance(KeyManagerFactory.getDefaultAlgorithm());
keyManagerFactory.init(keyStore, "keystore_pass".toCharArray());
sslContext.init(null, trustAllCerts, new SecureRandom());
client.sslSocketFactory(sslContext.getSocketFactory())
.hostnameVerifier(new HostnameVerifier() {
@Override
public boolean verify(String hostname, SSLSession session) {
return true;
}
});
Gson gson = new GsonBuilder().setLenient().create();
Retrofit retrofit = new Retrofit.Builder()
.baseUrl(Common.BASE_URL)
.addConverterFactory(GsonConverterFactory.create(gson))
.client(client.build())
.build();
serviceApi = retrofit.create(Api.class);
thanks hope this will help you. Sometime ssl version 1.2 or lower not installed in server side as well.
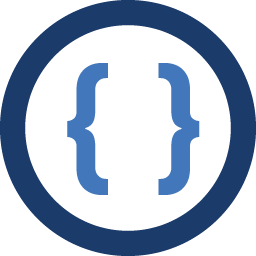
Author by
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
I'm using Retrofit 2.2.0 for uploading image to server (using Java). With an Android device (Samsung galaxy S6) API 24 (Build : NRD90M.G920FXXU5EQAC) when I try to post a request, this request failed with this error
javax.net.ssl.SSLHandshakeException: Handshake failed
ps: I try to downgrade the Retrofit 2.1.0 and it works perfectly.