Revalidating data using mutate in SWR - Which should I use?
Your code is almost correct, the issue lies in the fact that SWR
internally hashes the keys used for queries/mutation. Therefore, the key used by the mutate
function is simply not registered in the cache.
Using global mutate
To solve this problem using the mutate
function exported directly by swr
, it will suffice just to pass the same key to both useSWR
and mutate
.
mutate(["/api/albums/list?id=", appUser.id]);
This will invalidate the cache for the hashed key. The data retrieved from your original hook will go stale, and will therefore be fetched again.
Using bound mutate
Another option is to take advantage of the mutate
function bound to swr's key.
const { data, error, mutate } = useSWR(
["/api/albums/list?id=", appUser.id],
(url, params) => fetcher(url, params)
);
/*
* Now you can just call `mutate()` without specifying `key` argument
*/
docs: https://github.com/vercel/swr#bound-mutate
Specifying a refresh interval
For completeness, as you said, one other option would be just to set the value of refreshInterval
prop. Forcing a refetch when desired:
const { data, error } = useSWR(
["/api/albums/list?id=", appUser.id],
(url, params) => fetcher(url, params),
{
refreshInterval: 1000,
}
);
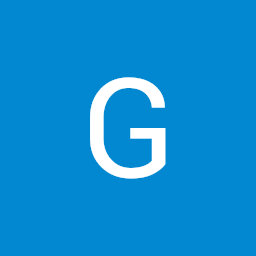
gabriel_tiso
Updated on June 09, 2022Comments
-
gabriel_tiso almost 2 years
In my app every time the user creates a new album the post request response responds with a list of the updated list of albums.
To provide a better user experience, I wanted the user to see the new content in the app without having to refresh the page.
I'm aware of the existence of SWR's mutate, but so far, I couldn't make it work.
I tried to set a 1000ms
refreshInterval
in my hook, but I wanted to know how to do it by using the mutate. Here's what I tried:SWR hook
const fetcher = async (url: string, param: string) => { const res = await fetch(url + param); return res.json(); }; const { data, error } = useSWR( ["/api/albums/list?id=", appUser.id], (url, params) => fetcher(url, params) );
Inside the
createAlbum
function:const data = await response.json(); mutate("/api/albums/list", data.newAlbums, false);
I would be happy to get some feedback.